PLC Libraries¶
Common Attributes and Functionality¶
Note
ESO PLC libraries have to be installed in the TwinCAT development environment before they can be used in projects.
Library content¶
For each function, e.g. lamp, shutter, motor, etc, the corresponding PLC library delivers:
- A function block (FB) for function control, e.g. FB_LAMP. Note that some libraries deliver more than one FB, e.g. Motor.library.
- A function block for the HW simulator, e.g. FB_SIM_LAMP
- A template GUI, e.g. GUI_TEMPLATE_LAMP
Input parameters¶
In most cases, apart from the Motor library, the configuration parameters can be given in the execution part, i.e. the FB call. This way some configuration parameters that are known that will not change, e.g. active low, timeouts, etc, can be ‘hard-coded’. Input parameters have the prefix in_. There is a mandatory input parameter called ‘in_sName’ that sets the name of the device. On PLC reboot these parameters will be set in the device configuration. However, the configuration defined in the Device Manager will overwrite the device configuration on INIT. This way, by giving input parameters the number of configuration parameters can be reduced or completely avoided but still with the flexibility of correcting any configuration parameter by the Device Manager without modifying the PLC code.
Example:
Lamp1(in_sName:='Lamp1', in_bActiveLowFault:=TRUE);
Interface with Device Manager¶
Device managers control the PLC device exclusively via RPC methods. There are a number of mandatory RPC methods that are common to all PLC devices. They are related to device state changes and logging. Each library, in addition to the function specific RPC methods, delivers the following RPCs:
- RPC_Init()
- RPC_Enable()
- RPC_Disable()
- RPC_SetDebug()
- RPC_SetLog()
- RPC_Stop()
- RPC_Reset()
In order to have RPC methods visible in the OPC UA address space, each declaration of an FB has to be coupled with a pragma statement {attribute ‘OPC.UA.DA’:=‘1’}.
Example:
{attribute 'OPC.UA.DA':='1'}
Lamp1: FB_LAMP;
{attribute 'OPC.UA.DA':='1'}
Lamp2: FB_LAMP;
Operational Logs at PLC Level¶
Device operations are by default logged on the PLC. The screenshot below shows how to open TwinCAT Logged Events Window.
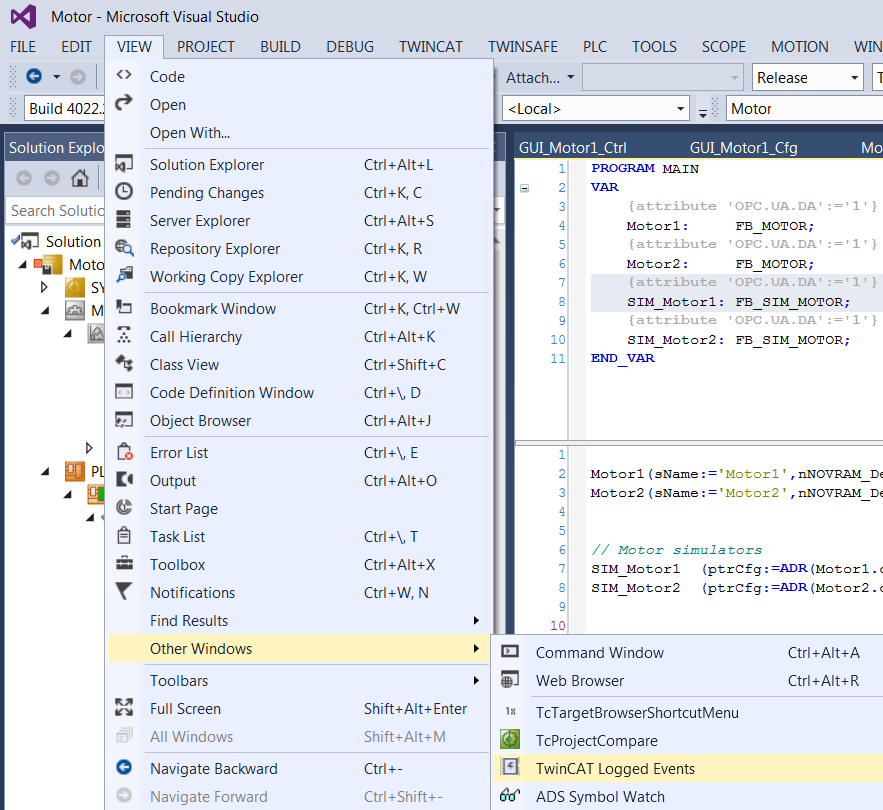
RPC methods RPC_SetDebug() and RPC_SetLog() are used to control the amount of logging information. If provided, debugging logs should be activated only for troubleshooting purpose since they could generate large amount of data.
In order to see the logs, the window has to be refreshed as shown below:
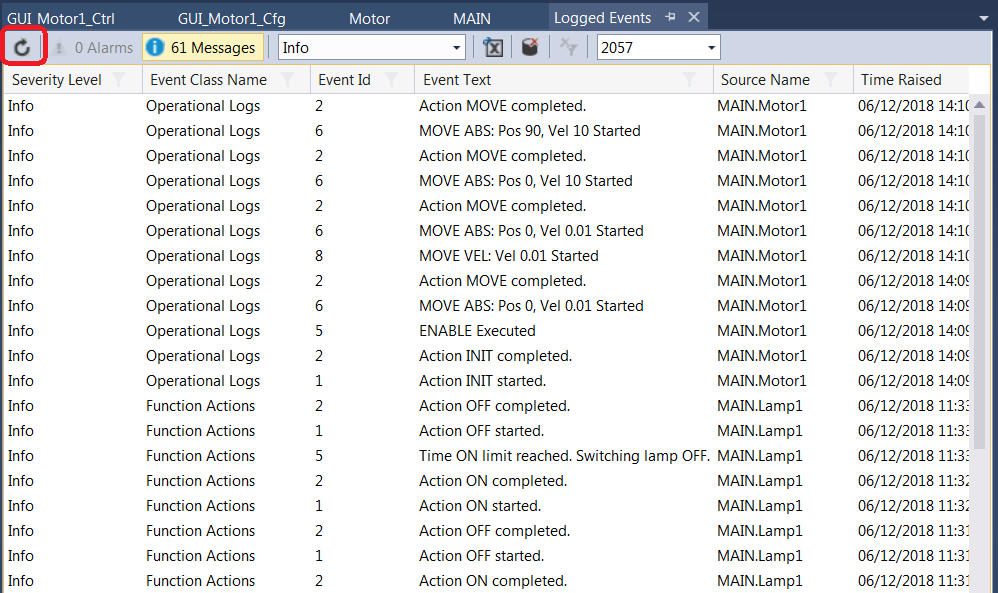
PLC simulators¶
In most cases the library includes a HW simulator that is linked to the device FB and gives quite realistic response. This way it is possible at a very early stage of the project to test Device Managers with a PLC. The device manager is not aware of the simulation at the PLC level and ‘believes’ that it communicates with a real HW.
Note
It is important to note that the PLC application, i.e. program MAIN, has to be modified in order to use simulators. In addition to the inclusion of a device simulator instance in MAIN, the device I/O variables have to be linked to the corresponding simulator I/O signals rather than to the real I/O.
HW simulators capture device INIT event and adjust their internal configuration ensuring that the device will work properly after the initialisation. For example, the simulator response time will be shorter than the device timeout.
Simulators provide RPC calls that are used to modify their response in order to test failure conditions of the device driver. For example, the Lamp RPC_SetFault() method can be used to set the fault signal of the lamp.
C++ Modules¶
For some PLC devices, additional software might be needed. TwinCAT enables the implementation of modules in C++ that run inside the TwinCAT real-time kernel. These modules communicate with the PLC via normal IO mapping (Communication between C++ modules and PLC).
The FCF provides some C++ modules for the computation of the field rotation that is used by tracking devices such as Derotators and ADCs devices.
The installation of the C++ module must be done on the computer that will be used to download and test PLC projects including tracking devices. These module has been already compiled and published by ESO and archived together with the Motor Library project.
Note
Visualisation and recompilation of the C++ module sources is only possible using commercial versions of Visual Studio (VS), e.g. VS Professional.
C++ modules can be imported in the standard TwinCAT environment without the need to recompile them. The procedure to import modules is described in the following link: (Importing C++ modules).
Tracking¶
Tracking controllers are motorized devices which update continuously the position of one or more motor axes as a function of the telescope coordinates, UTC time or variations of the temperature. Tracking controllers share some common characteristics which are described below.
- They support at least two modes of operations: one where motor axes are stationary and another one for tracking. In both cases the motor axes are moving in position mode.
- They may require an additional TwinCAT runtime license in case of using a C++ module. The required TwinCAT license is the C++ runtime.
- They may require a connection to the ELT time synchronization system to maximize tracking accuracy of the motor axes. A dedicated terminal (EL6688) is needed to connect to the ELT time synchronization system.
- The tracking position control loop is handled at lower level (PLC) by dedicated function blocks. The periodicity of the position control loop is configurable and does not depend on the PLC cycle.
The Motor Library provides two C++ modules that work together to compute the tracking data:
- TrkParams
- TrkModule
These two modules can be merged but in order to optimize the CPU is better to have them separated. The trkParams computes the parameters used by the slalib library and it does not require to be executed at a fast rate. The trkModule is the one computing the tracking data and is should be configured in a task running faster than the trkParams. It is suggested to use isolated CPUs to maximize performance as is shown in the figure below where one CPU is dedicated to the more demanding tasks.
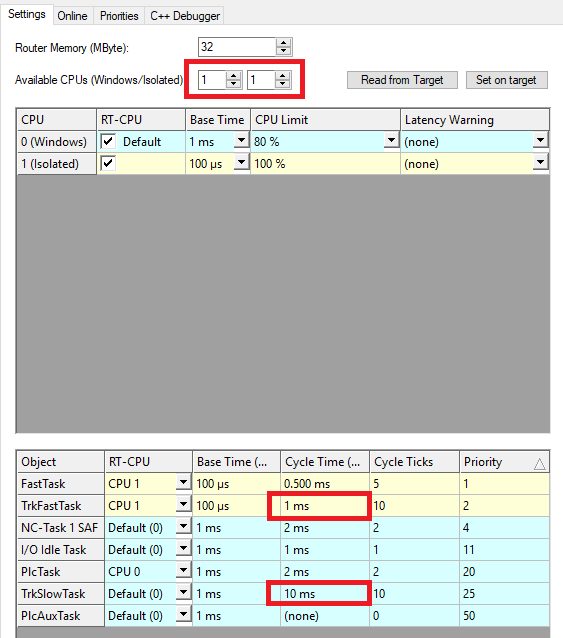
Suggestion for PLC CPU assignments.
Automatic TwinCAT Project Creation¶
A Windows utility called MakeProject.exe is provided for automatic creation of TwinCAT projects with a selectable number of motors, lamps and shutters. The utility generates a fully operational project that includes the selected number of devices and their simulators, i.e. every device is simulated at PLC level.
This can be very useful at very early stages of projects when HW is not available since it makes it possible to test the complete control system as if the HW were present. Once a particular piece of HW gets available, the corresponding simulator part should be removed from the project and the function block I/O variables linked to real system I/O.
The Windows executable can be downloaded from: http://svnhq9.hq.eso.org/p9/trunk/EELT/ICS/ifw/tools/twincat/makeTcProject/MakeProject.exe. There is no need to install the executable. It could be run from anywhere.
The utility is executed from a command line as shown below. In this particular case the executable was checked out into directory I:\Downloads.
The generated project is saved in directory C:\Temp\Solutions.
I:\Downloads>MakeProject.exe
Usage: MakeProject <name> <motors> <lamps> <shutters>
#
# Create a project called myProject with 5 motors, 3 lamps and 2 shutters.
#
I:\Downloads>MakeProject.exe myProject 5 3 2
Starting Visual Studio...
Creating empty project...
Populating project...
Adding libraries...
Building project...
Linking variables...
All done!
#
# The TwinCAT project is created in C:\Temp\Solutions
#
I:\Downloads>c:
C:\>cd Temp\Solutions
C:\Temp\Solutions>dir
Volume in drive C has no label.
Volume Serial Number is 1210-6BCB
Directory of C:\Temp\Solutions
12/12/2018 10:57 <DIR> .
12/12/2018 10:57 <DIR> ..
12/12/2018 10:54 <DIR> myProject
0 File(s) 0 bytes
3 Dir(s) 33,571,188,736 bytes free
C:\Temp\Solutions>dir myProject
Volume in drive C has no label.
Volume Serial Number is 1210-6BCB
Directory of C:\Temp\Solutions\myProject
12/12/2018 10:54 <DIR> .
12/12/2018 10:54 <DIR> ..
12/12/2018 10:53 <DIR> myProject
12/12/2018 10:54 4,340 myProject.sln
12/12/2018 10:54 116,281 myProject.tsproj
12/12/2018 10:54 111,456 myProject.tsproj.bak
12/12/2018 10:54 <DIR> _Boot
12/12/2018 10:54 <DIR> _Config
3 File(s) 232,077 bytes
5 Dir(s) 33,571,188,736 bytes free
Lamp Library (Lamp.library)¶
FB_LAMP is the TwinCAT PLC Function Block to operate the low level control of a standard lamp device with or without intensity control.
Input parameters¶
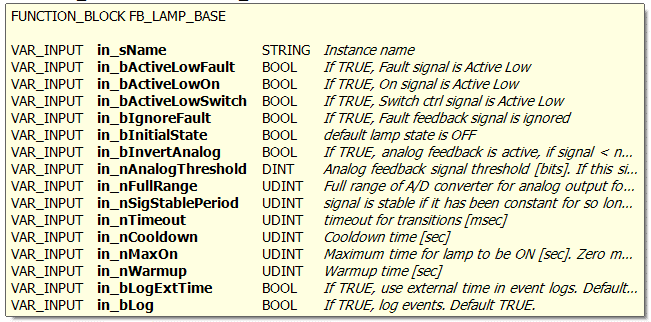
Signal Mapping¶
The figure below shows the TwinCAT view of the FB_LAMP I/O variables that are available for mapping to physical signals, i.e. ports of I/O terminals.
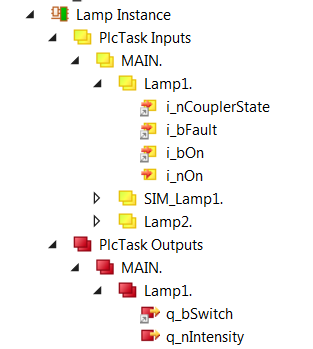
Example of a FB_LAMP input/output signals.
The table below describes each mapping variable.
Variable | Port Type | Optional Mapping | Description |
---|---|---|---|
i_nCouplerState | UINT | No | Mapped to the ‘state’ of the coupler that hosts I/O terminals. If the terminals span over more than one coupler, it is recommended to select the ‘state’ of the last coupler that hosts a lamp signal. |
i_bFault | Digital In | Yes | Does not have to be mapped if ‘fault’ signal is ignored, i.e. does not exist. |
i_bOn | Digital In | No | Mapped to the Lamp status (ON/OFF) digital input signal. |
i_nOn | Analog In | Yes | Analog feedback signal. Has to be mapped only if analog feedback is used. |
q_bSwitch | Digital Out | No | Mapped to the Lamp control digital output signal. |
q_nIntensity | Analog Out | Yes | Mapped to the Lamp intensity analog output signal (if exists). |
GUI Template¶
The Lamp Library provides a template GUI to control an FB_LAMP. Applications can easily deploy an instance of this GUI to control their own lamp function blocks by setting the GUI references to the particular instance of FB_LAMP, as shown below.

Instantiation of GUI_TEMPLATE_LAMP for Lamp1
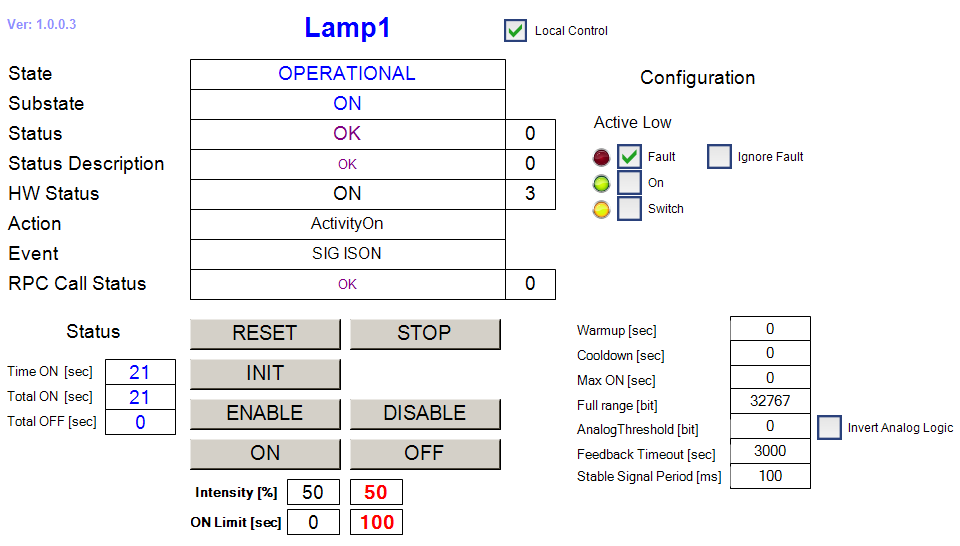
FB_LAMP HMI for Local Control.
Lamp specific RPC Methods¶
- RPC_Off() Turn lamp OFF
- RPC_On() Turn lamp ON
Lamp Simulator¶
The function block FB_SIM_LAMP implements the lamp simulator on the PLC. The simulator has the address of the lamp instance as the only input parameter. The following code shows how the simulator is declared and executed:
Declaration:
{attribute 'OPC.UA.DA':='1'}
Lamp1: FB_LAMP; // Simulated lamp
{attribute 'OPC.UA.DA':='1'}
SIM_Lamp: FB_SIM_LAMP; // Lamp simulator
Execution:
Lamp1(in_sName:='Lamp1',in_bActiveLowFault:=TRUE,);
SIM_Lamp(ptrDev:=ADR(Lamp1));
Simulator Mapping¶

Simulator RPC Methods¶
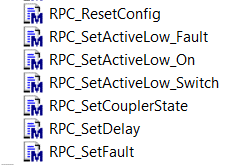
Shutter Library (Shutter.library)¶
FB_SHUTTER is the TwinCAT PLC Function Block to operate the low level control of a standard shutter device.
Input parameters¶
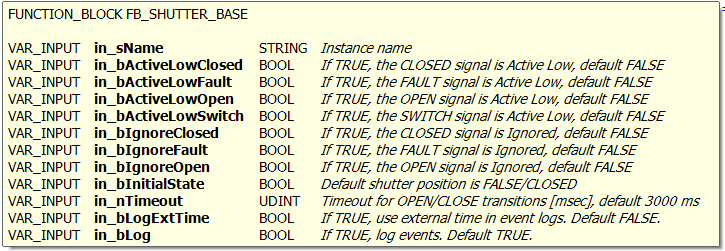
Signal Mapping¶
The figure below shows the TwinCAT view of the FB_SHUTTER I/O variables that are available for mapping to physical signals, i.e. ports of I/O terminals.
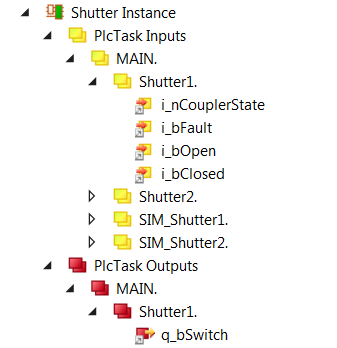
Figure 4: Example of a FB_SHUTTER input/output signals.
The table below describes each mapping variable.
Variable | Port Type | Optional | Description |
---|---|---|---|
i_nCouplerState | UINT | No | Mapped to the ‘state’ of the coupler that hosts I/O terminals. If the terminals span over more than one coupler, it is recommended to select the ‘state’ of the last coupler that hosts a shutter signal. |
i_bFault | Digital In | Yes | Does not have to be mapped if ‘fault’ signal is ignored, i.e. does not exist. |
i_bOpen | Digital IN | No | Mapped to the Shutter ‘open’ digital input signal. |
i_bClosed | Digital IN | No | Mapped to the Shutter ‘closed’ digital input signal. |
q_bSwitch | Digital Out | No | Mapped to the Shutter control digital output signal. |
GUI Template¶
The Shutter Library provides a template GUI to control an FB_SHUTTER. Applications can easily deploy an instance of this GUI to control their own shutter function blocks by setting the GUI references to the particular instance of FB_ SHUTTER, as shown below.

Figure 5: Instantiation of GUI_TEMPLATE_SHUTTER for Shutter1
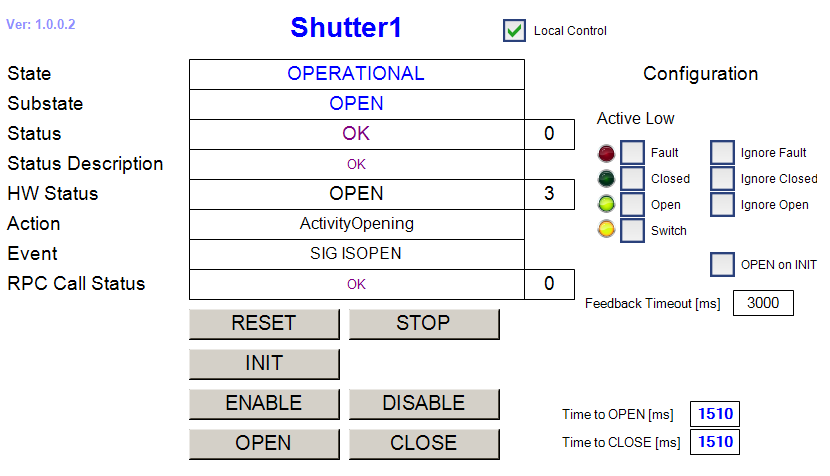
Figure 6: FB_SHUTTER HMI for Local Control.
Shutter specific RPC Methods¶
- RPC_Close() Close shutter
- RPC_Open() Open Shutter
Shutter Simulator¶
The function block FB_SIM_SHUTTER implements the shutter simulator on the PLC. The simulator has the address of the shutter instance as the only input parameter. The following code shows how the simulator is declared and executed:
Declaration:
{attribute 'OPC.UA.DA':='1'}
Shutter1: FB_SHUTTER;
{attribute 'OPC.UA.DA':='1'}
SIM_Shutter1: FB_SIM_SHUTTER;
Execution:
Shutter1(in_sName:='Shutter1', in_bActiveLowOpen:=TRUE, in_bActiveLowClosed:=TRUE);
SIM_Shutter1(ptrDev := ADR(Shutter1));
Simulator Mapping¶
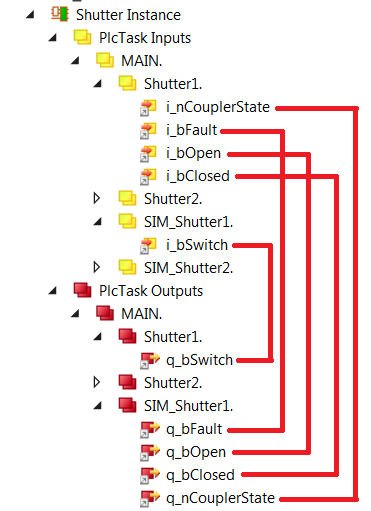
Simulator RPC Methods¶
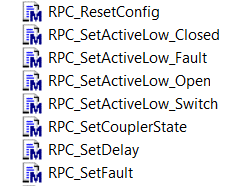
Time Library (Timer.library)¶
FB_TIME is a simple ESO PLC Function Block. This FB aims to provide time services to other components running within the PLC, e.g. computation of absolute time.
Input parameters¶
The FB_TIME does not have input parameters.
Signal Mapping¶
The FB_TIME defines a number of mappings that are needed to do the correct computation of the time. Most of these mappings come from the EL6688 terminal. If this terminal is not available in the HW configuration, the FB_TIME could still be used to simulate a particular time in the PLC.
The table below describes each mapping variable.
Variable | Port Type | Optional Mapping | Description |
---|---|---|---|
i_inTime | ULINT | No | Mapped to the EL6688 internal time stamp |
i_exTime | ULINT | No | Mapped to the EL6688 external time stamp |
i_dc2TcOffset | LINT | No | Mapped to the EtherCAT Dc2TcOffet |
i_dc2ExtOffset | LINT | Yes | Mapped to the EtherCAT Dc2ExtOffset |
i_extDevNotConnected | BOOL | No | Mapped to the EL6688 External device not connected |
i_syncMode | UINT | Yes | Mapped to the EL6688 Sync mode |
i_AdsAddr | AMSADDR | No | Mapped to the EL6688 ADS address |
q_timeInfo | T_TIME_INFO | Yes | Delivers the actual absolute time (DC) and mode |
GUI Template¶
As for the other PLC libraries, the Timer Library provides a template GUI to control the FB_TIME. Applications can easily deploy an instance of this GUI to control their own time function block by setting the GUI references to the particular instance of FB_TIME.
Instantiation of GUI_TEMPLATE_LAMP for time_info

FB_TIME HMI for Local Control.
Time specific RPC Methods¶
- RPC_GetMode() Get actual time mode: Local, UTC or Simulation.
- RPC_SetMode() Set new time mode (Local, UTC or Simulation).
- RPC_GetUTC() Obtain the actual absolute time and mode.
- RPC_SetTime(string) Set a user defined time (only works in Simulation mode).
Motor Library (Motor.library)¶
Warning
The Motor.library provides a number of function blocks related to motion control. For the Alpha release only discrete motion (FB_MOTOR) is fully supported and described in this document. Users should not use other FBs found in the library without consultation with ESO.
FB_MOTOR is the TwinCAT PLC Function Block used to operate motors. The library is based on the Beckhoff MC Library that is in turn PLCOpen MC compliant. This means that it should be possible to use the library for control of any type of motor, including brushless motors, under the condition that the motor controller is fully EtherCAT certified. So far, stepper, DC and synchronous motors have been tested with the library.
Usage of NOVRAM¶
NOVRAM is used to store and keep the configuration of the motor. The configuration of the motor is copied into the NOVRAM on every successful INIT of the motor. On power cycle of the PLC, the configuration of the motor is read from the NOVRAM during the first PLC cycle. As a general rule, only PLCs with NOVRAM should be used for motor control applications, e.g. CX-2030 that comes with 128 kB of NOVRAM. PLCs without NOVRAM can still be used for motor control applications but the configuration will be lost on every power cycle of the PLC. These applications have to rely on the higher level software that has to download the configuration after each power cycle. The other option is to hard-code the configuration in the PLC application itself.
Input parameters¶

FB_MOTOR has three input parameters. Apart from the standard sName parameter for the name, i.e. the label, of the motor instance, there are two additional parameters related to the usage of the NOVRAM.
Parameter | Type | Description |
---|---|---|
sName | STRING | Motor instance name/label |
nNOVRAM_DevId | UDINT | NOVRAM Device ID. For PLCs without NOVRAM, this parameter should be set to zero or not given at all. |
nNOVRAM_Offset | UDINT | Offset in bytes in NOVRAM. A motor instance needs about 500 bytes for configuration data. For simplicity it is recommended to increment offsets by 1000 for each motor. Therefore, the first motor will have the offset of zero, second of 1000, third of 2000, etc. For PLCs without NOVRAM, this parameter should be set to zero or not given at all. |
The following example shows the usage of NOVRAM. It is very important to note that the entered value for the parameter nNOVRAM_DevId matches the value of the NOV-DP-RAM device. In this example the value is 4.
In case that the NOVRAM is not used, the Motor1 instance would not have any parameters and the code would look like this:
Motor1(sName:=’Motor1’);
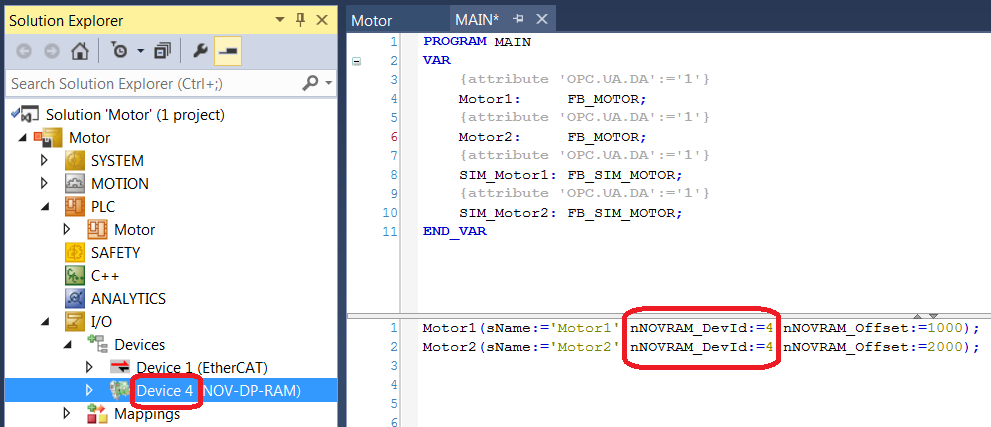
Figure 7: Application example for using NOVRAM with FB_MOTOR.
Signal Mapping¶
Figure 8 shows the TwinCAT view of the FB_MOTOR I/O variables that are available for mapping to physical signals, i.e. ports of I/O terminals or NC structures.
The mapping is quite different from other devices. Specific to motors, for each Axis (i.e. motor) there are two links to be established from the Axes/<Motor> Settings:
- Link To I/O… This is the link between the Axis and the motor controller terminal, e.g. EL7041, that controls it.
- Link To PLC… This is the link between the Axis and the motor instance in the MAIN program, e.g. Motor1. This link sets all the mappings for the two complex structures NcToPlc (input) and PlcToNc (output) and the user should not touch it afterwards.
The mapping parameters are described in the table below.
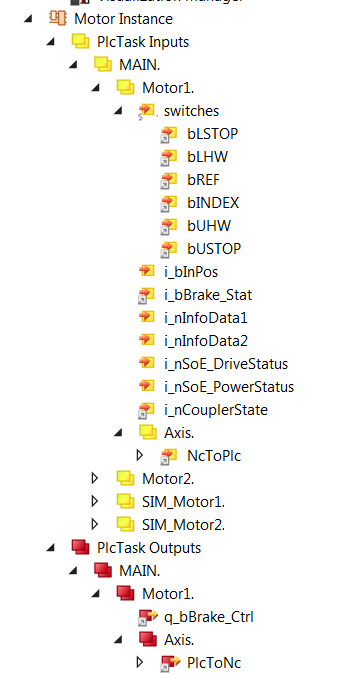
Figure 8: Example of a FB_MOTOR input/output signals.
Variable | Port Type | Optional | Description |
---|---|---|---|
switches | Digital In | Yes | There are six variables to be linked to various limit and reference switches, depending on the HW configuration; upper and lower Stop and HW limits, Reference and Index switch. Switches can be linked to any binary signal (BOOL). Normally the signals are coming from digital inputs but they could also be linked to variables of type BOOL in some special applications, i.e. digitizing of analog position sensors. |
i_bInPos | Digital In | Yes | Signal of the In-Position switch. In some applications with stepper motors without feedback (cryogenic environment) a switch is used to confirm that the motor is in correct position. |
i_bBrakeStat | Digital In | Yes | Status of the brake feedback signal. |
i_nInfoData1/2 | INT | Yes | Two freely selectable signed integers to be link to any variable of the same type, e.g. motor controller output current. |
i_nSoE_DriveStatus | UINT | Yes | Serco Drive (e.g. AX5000) Status, not used with CoE drives. |
i_nSoE_PowerStatus | UINT | Yes | Serco Drive (e.g. AX5000) Power Status, not used with CoE drives. |
i_nCouplerState | UINT | No | Mapped to the ‘state’ of the coupler that hosts I/O terminals. If the terminals span over more than one coupler, it is recommended to select the ‘state’ of the last coupler that hosts a shutter signal. |
NcToPlc | Struct In | No | Internal MC structure of type MC.NCTOPLC_AXIS_REF. Automatically linked from “Link To PLC”. |
q_bBrake_Ctrl | Digital Out | Yes | Brake control digital output signal. Active low! |
PlcToNc | Struct Out | No | Internal MC structure of type MC.PLCTONC_AXIS_REF. Automatically linked from “Link To PLC”. |
GUI Templates¶
The Motion Library provides two template GUIs intended for configuration and control of motor instances of FB_MOTOR respectively. Applications can easily deploy instances of the GUIs by setting the GUI references to the particular instance of FB_MOTOR_CONTROL, as shown below for the case of the configuration GUI.
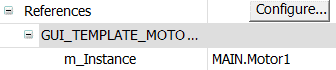
Figure 9: Instantiation of GUI_TEMPLATE_MOTOR_CONFIG for Motor1
From the Configuration GUI, shown on Figure 10, it is very simple to set axis type, define INIT sequence, select user defined methods to be executed, set the active low parameters for each switch, as well as to configure brakes, define timeouts, software limits and backlash compensation.
Once the motor is successfully initialised, all configuration will be stored in NOVRAM.
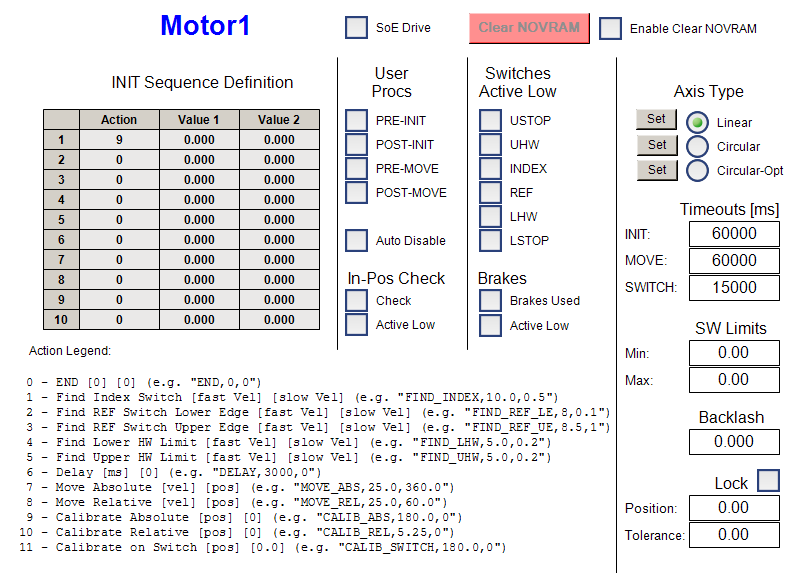
Figure 10: FB_MOTOR HMI for motor configuration.
From the Control GUI, shown on Figure 11, it is possible to perform basic control operations on the motor.
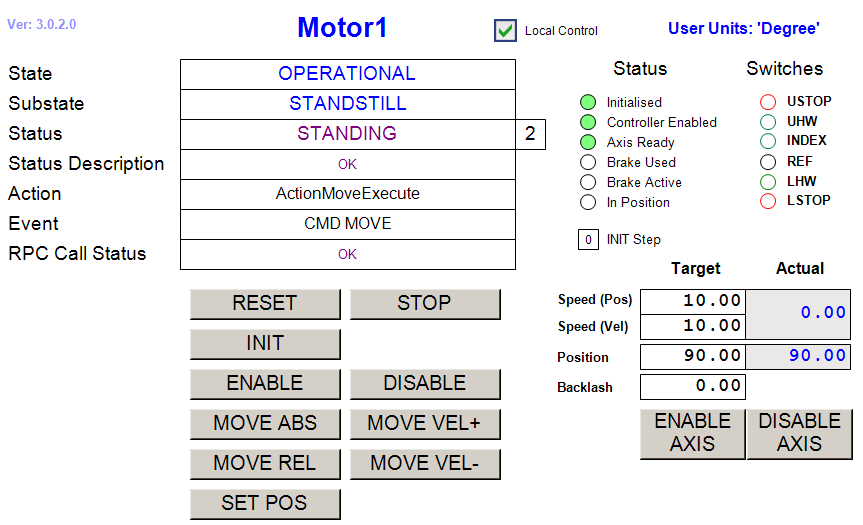
Figure 11: FB_MOTOR HMI for Local Control.
Motor specific RPC Methods¶
- RPC_MoveAbs() Move to absolute position
- RPC_MoveRel() Move relative to current position
- RPC_MoveVel() Move in velocity
Motor Simulator¶
Note
Important note: In order to use motor simulator, the Axis has to be modified. The Axis Type has to be set to Standard (Mapping via Encoder and Drive) and the Link to I/O… should be left empty. The Axis Encoder has to be configured as Simulation encoder. See screen shots below.

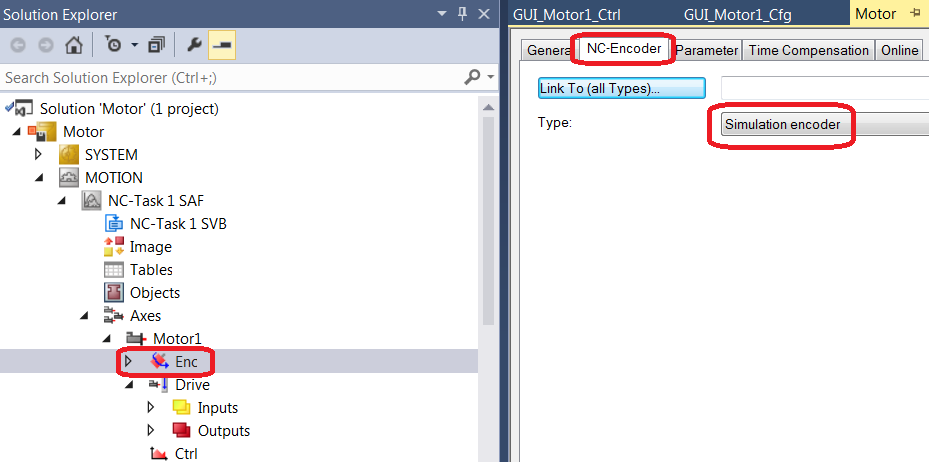
The function block FB_SIM_MOTOR implements the motor simulator on the PLC. The input parameter of the simulator are addresses of the motor instance configuration and status structures. The following code shows how the simulator is declared and executed:
Declaration:
{attribute 'OPC.UA.DA':='1'}
Motor1: FB_MOTOR;
{attribute 'OPC.UA.DA':='1'}
SIM_Motor1: FB_SIM_MOTOR;
Execution:
Motor1(sName:='Motor1',nNOVRAM_DevId:=0);
SIM_Motor1 (ptrCfg:=ADR(Motor1.cfg), ptrStat:=ADR(Motor1.stat));
Simulator Mapping¶
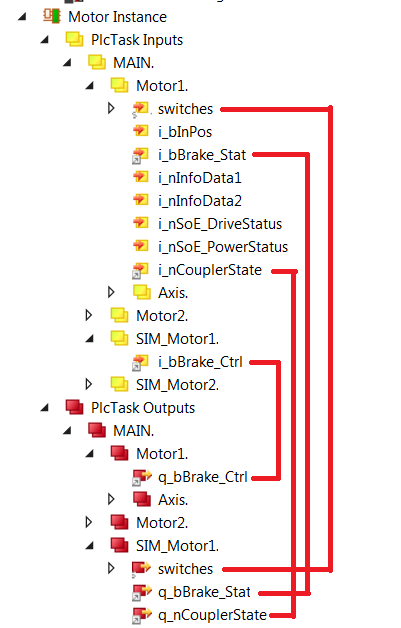
Simulator RPC Methods¶
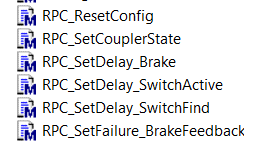
User Defined Methods¶
The functionality of FB_MOTOR can be extended by user defined Pre- and Post INIT and MOVE methods. FB_MOTOR already includes the four dummy methods (see below) that only add a delay of three seconds during the execution. However, the dummy implementation can be a good starting point when overloading these methods for specific implementations in FBs that extend FB_MOTOR functionality.
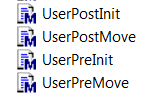
CCS Simulator (Motor.library)¶
Tracking devices require information from CCS to be able to compute the corrections to be applied to the actuators. This information will be obtained later from CCS but in the meantime, FCF includes a utility that enable a simple way to validate tracking without the CCS infrastructure. It is a dummy FB (FB_CCS_SIM) that can be edited to define the telescope coordinates, environmental conditions and other parameters needed by the library computing the tracking data (slalib). This FB avoid duplicating this information per each tracking device.
Input parameters¶
The FB_CCS_SIM does not have input parameters.
Signal Mapping¶
Figure below shows an example of the mapping related to the CCS Simulator (ccs_sim). It relates to the C++ modules, an instance to the FB_TIME (time_info) and a tracking device such a the derotator (drot).
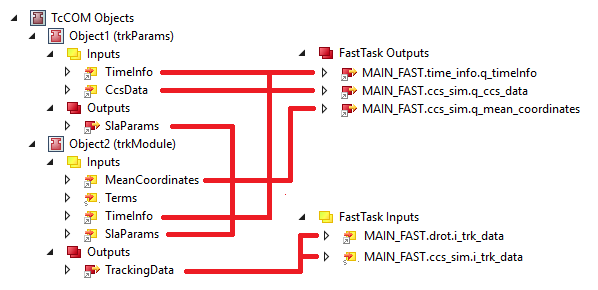
Example of the FB_CCS_SIM mapping.
The specific mapping parameters of CCS Simulator are described in the table below.
Variable | Port Type | Optional Mapping | Description |
---|---|---|---|
i_trk_data | TrackingData | No | Structure delivered by the C++ Module |
q_ccs_data | CcsData | No | Structure containing the complete CCS data |
q_mean_coordinates | TrkMeanCoordinates | No | Structure containing the MEAN coordinates |
GUI Template¶
As for the other FBs, the Motor Library provides a template GUI to control the FB_CCS_SIM. Applications can easily deploy an instance of this GUI to control their own time function block by setting the GUI references to the particular instance of FB_CCS_SIM.
Instantiation of GUI_TEMPLATE_CCS_SIM for ccs_sim
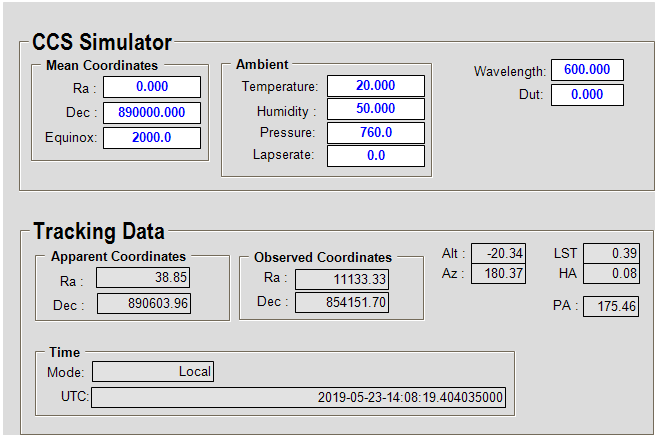
FB_CCS_SIM HMI for Local Control.
CCS_SIM specific RPC Methods¶
RPC_SetCoordinates() This RPC allows the user to change RA,DEC and EQUINOX via OPCUA.
Drot Controller (Motor.library)¶
FB_MA_DROT is the TwinCAT PLC Function Block used to operate a derotator. The FB encapsulates the motion control functionality using a composition of a motor device together with the functionality to derive the next motor position using the field rotation obtained from a dedicated C++ module.
Note
All the functionalities provided for a normal motor device are available as well for the derotator.
Input parameters¶
FB_MA_DROT has four input parameters. Apart from the standard sName parameter for the name, i.e. the label, of the derotator instance, there are three additional parameters used by the motor used internally by the derotator.
Parameter | Type | Description |
---|---|---|
sName | STRING | Drot instance name/label |
sMotorName | STRING | Motor instance name/label |
nNOVRAM_DevId | UDINT | NOVRAM Device ID. For PLCs without NOVRAM, this parameter should be set to zero or not given at all. |
nNOVRAM_Offset | UDINT | Offset in bytes in NOVRAM. A motor instance needs about 500 bytes for configuration data. For simplicity it is recommended to increment offsets by 1000 for each motor. Therefore, the first motor will have the offset of zero, second of 1000, third of 2000, etc. For PLCs without NOVRAM, this parameter should be set to zero or not given at all. |
Note
The usage of NOVRAM follows the same guidelines as for a FB_MOTOR.
In case that the NOVRAM is not used, the Drot1 instance would not have any parameters and the code would look like this:
Motor1(sName:=’Drot1’, sMotorName:= 'Drot1Motor');
Signal Mapping¶
The mapping includes all the mapping of a motor plus the specific mapping of the derotator.
Specific to the internal derotator motor, there are two links to be established from the Axes/<Motor> Settings:
- Link To I/O… This is the link between the Axis and the motor controller terminal, e.g. EL7041, that controls it.
- Link To PLC… This is the link between the Axis and the motor instance in the MAIN program, e.g. drot1.motor. This link sets all the mappings for the two complex structures NcToPlc (input) and PlcToNc (output) and the user should not touch it afterwards.
The description of the standard motor mapping can be found here motor-signal-mapping
The additional derotator mapping parameters are described in the table below.
Variable | Port Type | Optional | Description |
---|---|---|---|
i_trk_data | Struct In | No | Tracking data computed by the C++ module. |
i_ccs_data | Struct In | Yes | CCS Simulation data |
Warning
The above mapping table does not include the standard motor mapping.
GUI Templates¶
The Motion Library provides one template GUI intended for the control of instances of FB_MA_DROT. Applications can easily deploy instances of the GUI by setting the GUI reference to the particular instance of FB_MA_DROT.
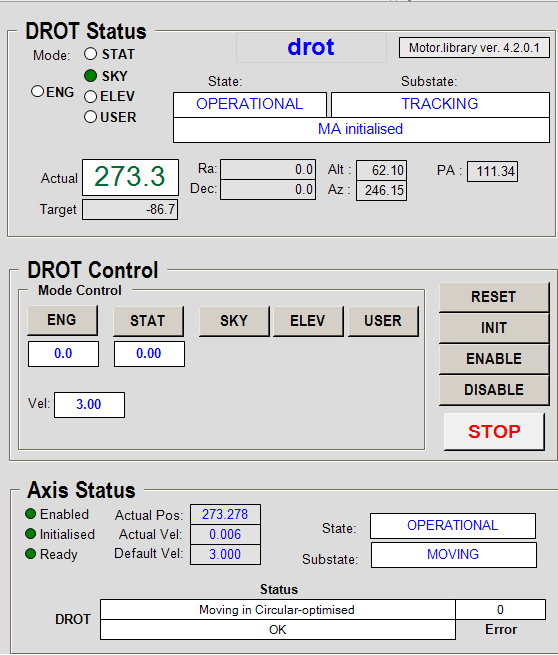
FB_MA_DROT HMI for Local Control
From the Derotator Control GUI, shown above, it is possible to perform basic control operations on the Derotator. The GUI also shows the status of the subordinated motor.
Note
It is possible to create dedicated control and configuration GUIs for the derotator subordinated motor as for any other motor device.
Derotator specific RPC Methods¶
RPC name | Parameters | Description |
---|---|---|
RPC_MoveAngle() | in_lrAngle | Move the derotator to a particular position angle. |
RPC_StartTrack() | in_mode in_angle | Start derotator tracking. |
RPC_StopTrack() | Stop derotator tracking. |
Derotator Simulator¶
Derotator does not have a dedicated simulator. Motor simulator shall be used to simulate derotator behaviour.
Declaration:
{attribute 'OPC.UA.DA':='1'}
drot: FB_MA_DROT;
{attribute 'OPC.UA.DA':='1'}
sim_drot: FB_SIM_MOTOR;
Execution:
drot(sName:='DROT', sMotorName:= 'DrotMotor', nNOVRAM_DevId:=0);
sim_drot(ptrCfg:=ADR(drot.motor.cfg), ptrStat:=ADR(drot.motor.stat));
User Defined Methods¶
Derotator user defined functions are the same as for the Motor.
ADC Controller (Motor.library)¶
FB_MA_ADC is the TwinCAT PLC Function Block used to operate a ADC. The FB encapsulates the motion control functionality using a composition of two internal motors (motor1 and motor2).
Note
All the functionalities provided for a normal motor device are available as well for the internal ADC motors.
Input parameters¶
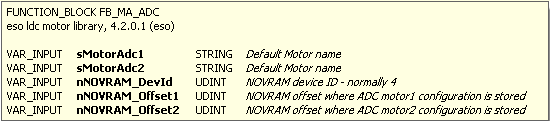
FB_MA_ADC has five input parameters as shown in figure above.
Note
The usage of NOVRAM follows the same guidelines as for a FB_MOTOR.
Signal Mapping¶
The mapping includes all the mapping of the two internal motors plus the specific mapping of the ADC.
Specific to the internal ADC motors, there are two links to be established from each of the Axes/<Motor> Settings:
- Link To I/O… This is the link between the Axis and the motor controller terminal, e.g. EL7041, that controls it.
- Link To PLC… This is the link between the Axis and the motor instance in the MAIN program, e.g. drot1.motor. This link sets all the mappings for the two complex structures NcToPlc (input) and PlcToNc (output) and the user should not touch it afterwards.
The description of each standard motor mapping can be found here motor-signal-mapping
The additional ADC mapping parameters are described in the table below.
Variable | Port Type | Optional | Description |
---|---|---|---|
i_trk_data | Struct In | No | Tracking data computed by the C++ module. |
i_ccs_data | Struct In | Yes | CCS Simulation data |
Warning
The above mapping table does not include the mapping for the two internal motors.
GUI Templates¶
The Motion Library provides one template GUI intended for the control of instances of FB_MA_ADC. Applications can easily deploy instances of the GUI by setting the GUI reference to the particular instance of FB_MA_ADC.
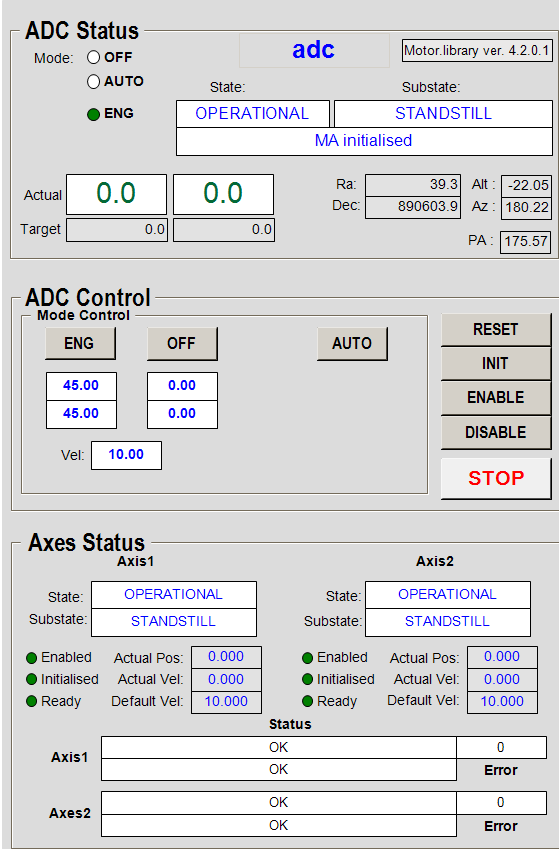
FB_MA_ADC HMI for Local Control
From the ADC Control GUI, shown on above, it is possible to perform basic control operations on the ADC. The GUI also shows the status of the subordinated motors.
Note
It is possible to create dedicated control and configuration GUIs for the ADC subordinated motors as for any other motor device.
ADC specific RPC Methods¶
RPC name | Parameters | Description |
---|---|---|
RPC_MoveAngle() | in_lrAngle | Move the ADC to a particular position angle. |
RPC_StartTrack() | in_angle | Start ADC tracking. |
RPC_StopTrack() | Stop ADC tracking. |
ADC Simulator¶
ADC does not have a dedicated simulator. Motor simulator shall be used to simulate the behaviour of the two internal motors.
Declaration:
{attribute 'OPC.UA.DA':='1'}
adc: FB_MA_ADC;
{attribute 'OPC.UA.DA':='1'}
sim_adc1: FB_SIM_MOTOR;
{attribute 'OPC.UA.DA':='1'}
sim_adc2: FB_SIM_MOTOR;
Execution:
adc(sName:='ADC',
sMotorAdc1:= 'AdcMotor1',
sMotorAdc2:= 'AdcMotor2',
nNOVRAM_DevId:=4,
nNOVRAM_Offset1:=3000,
nNOVRAM_Offset2:=4000);
sim_adc1(ptrCfg:=ADR(adc.motor1.cfg), ptrStat:=ADR(adc.motor1.stat));
sim_adc2(ptrCfg:=ADR(adc.motor2.cfg), ptrStat:=ADR(adc.motor2.stat));
User Defined Methods¶
No user defined functions are provided by the ADC.
I/O Device Library (IODev.library)¶
IODev.library contains a number of FBs for handling analog and digital sensors and I/O devices. In addition, it provides FB methods for scaling of signals in order to work directly in user units (temperatures, pressures, etc), instead of displaying pure voltages that cannot be easily interpreted by the user before they are scaled at the WS level. Simulators for analog and digital signals are also provided. They might be of great help at the early stages of projects when sensors are not available yet or for testing of out‑of‑range conditions.
The library provides a Function Block called FB_IODEV_BASE that has to be extended and customised by the user by adding input and output signals to the status and control structures. A number of I/O and Sensor FB examples that extend the FB_IODEV_BASE functionality can be found in the Examples directory. Example FBs with the string ‘_USER_CONFIG’ in their name provide examples of user defined configuration, i.e. signal scaling, that is implemented in the M_UserConfigure() method. In addition to the extension of the FB functionality, the user has to extend the definition of the status T_IODEV_STAT and control T_IODEV_CTRL structures (if any) by adding arrays of specific input and output signals.
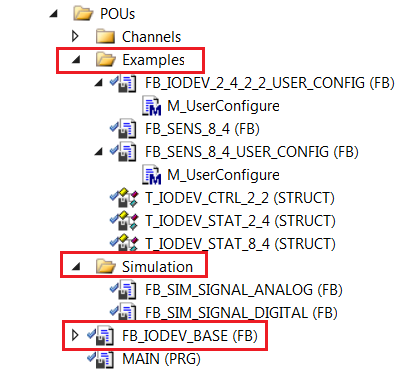
Overview of IODev.library
Input parameters¶

User Customisation¶
As previously stated, the Function Block FB_IODEV_BASE provides all methods to handle I/O signals but no signals are defined. The FB has to be extended by the user by adding required I/O signals. The customisation process will be described through examples.
Example 1: I/O Device with User Defined Scaling¶
The FB FB_IODEV_2_4_2_2_USER_CONFIG is an example of the I/O device that handles 2 DI, 4 AI, 2 DO and 2 AO, with the user defined scaling.
The following steps have to be done:
- Extend the T_IODEV_STAT structure by adding arrays of input signals. The new structure is called T_IODEV_STAT_2_4, meaning 2 digital inputs and 4 analog inputs.
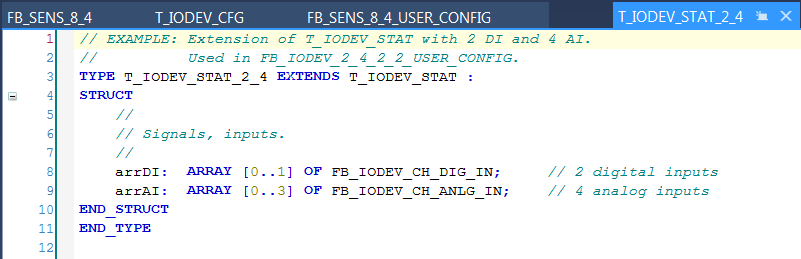
- Extend the T_IODEV_CTRL structure by adding arrays of output signals. The new structure is called T_IODEV_CTRL_2_2, meaning 2 digital outputs and 2 analog outputs.
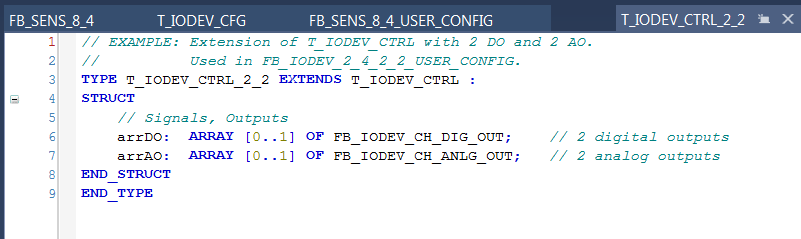
- Define signal scaling for both inputs and outputs.
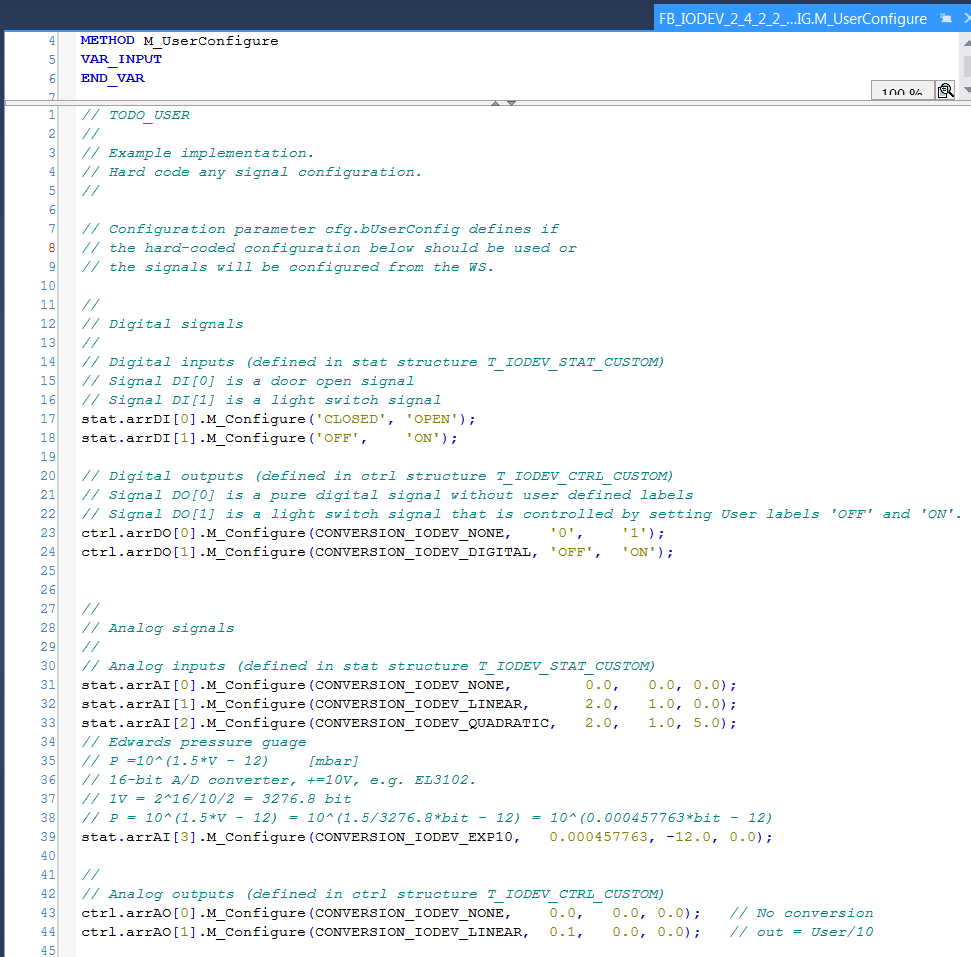
Extend the functionality of FB_IODEV_BASE in newly created FB FB_IODEV_2_4_2_2_USER_CONFIG.
The code below shows all that the user has to do in order to define a FB that extends the functionality of FB_IODEV_BASE. The following has to be done in the FB:
- In the declaration of FB FB_IODEV_2_4_2_2_USER_CONFIG, add ctrl and stat structures. They should be of type T_IODEV_CTRL_2_2 and T_IODEV_STAT_2_4, respectively.
- Set references to ctrl and stat structures.
- Set the number of existing I/O signals.
- Set the pointers to the first item of each signal array.
- Execute the code of the base (SUPER) FB.
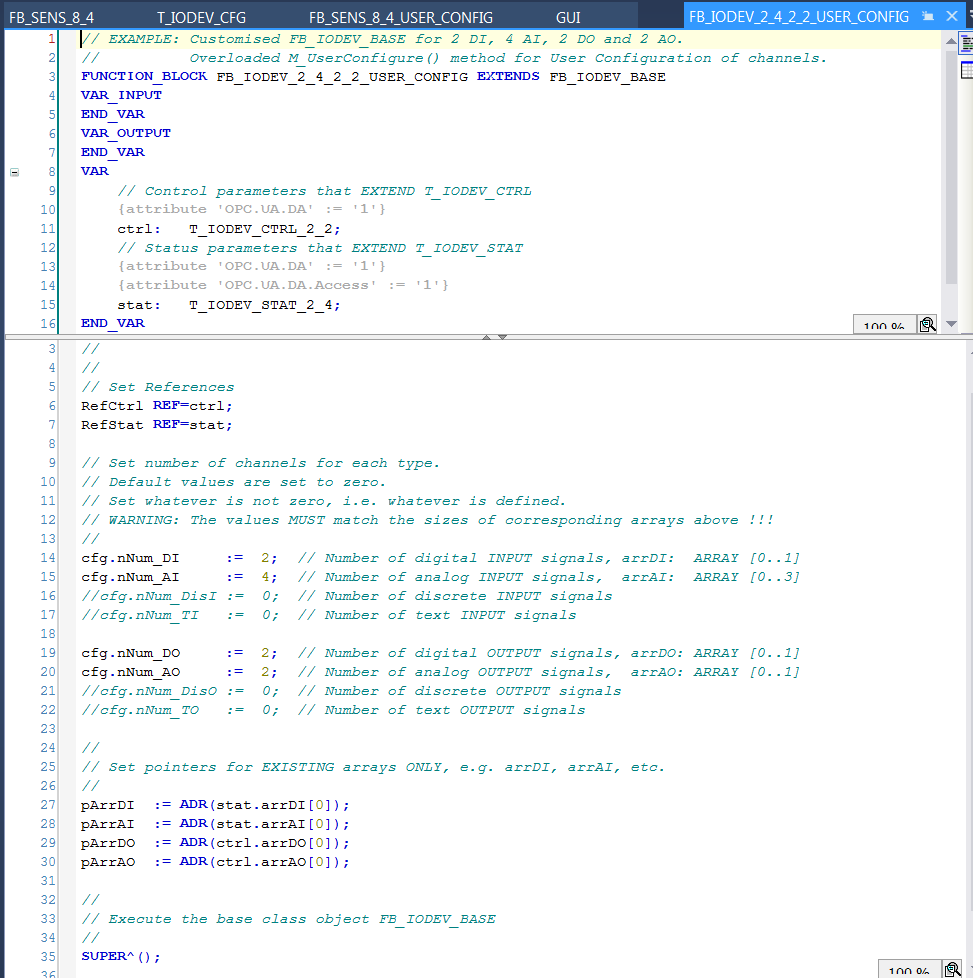
Example 2: Sensor Device without User Defined Scaling¶
The Function Block FB_SENS_8_4 is an example of a sensor device for eight digital and four analog input signals. The corresponding status data structure is called T_IODEV_STAT_8_4. Since there are no output signals, the standard control structure T_IODEV_CTRL is used. In this example user scaling is not used, so the scaling is supposed to be done on the WS.
The following steps have to be done:
- Extend the T_IODEV_STAT structure by adding arrays of input signals. The new structure is called T_IODEV_STAT_8_4, meaning 8 digital inputs and 4 analog inputs.
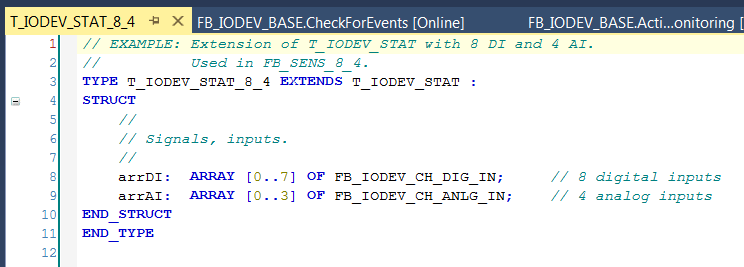
- Create FB FB_IODEV_2_4_2_2_USER_CONFIG and extend the functionality of FB_IODEV_BASE as shown below.
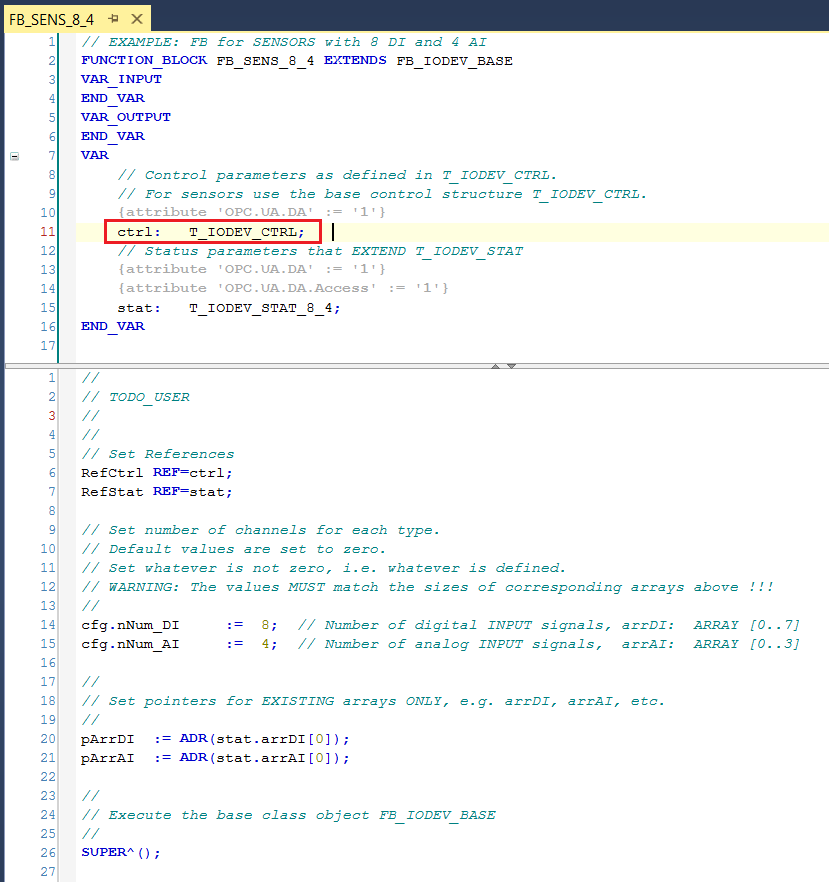
Signal Mapping¶
As an example, the figure below shows the TwinCAT view of the FB_IODEV_2_4_2_2_USER_CONFIG I/O variables that are available for mapping to physical signals, i.e. ports of analog and digital I/O terminals.
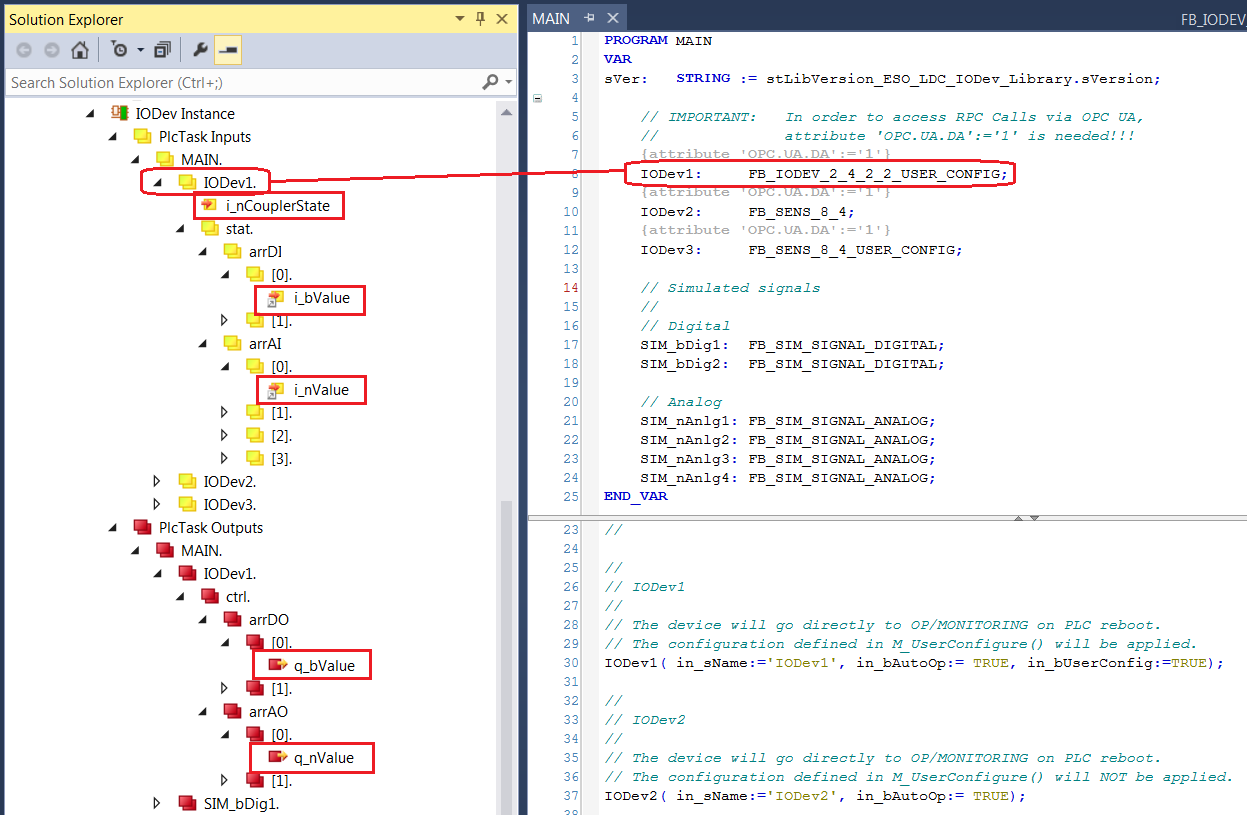
Figure 12: Example of a FB_IODEV_2_4_2_2_USER_CONFIG signals.
The table below describes each mapping variable.
Variable | Port Type | Optional | Description |
---|---|---|---|
i_nCouplerState | UINT | No | Mapped to the ‘state’ of the coupler that hosts I/O terminals. If the terminals span over more than one coupler, it is recommended to select the ‘state’ of the last coupler that hosts a shutter signal. |
arrDI[i].i_bValue | BOOL | Yes | Array of digital input signals |
arrAI[i].i_nValue | INT | Yes | Array of analog input signals |
arrDO[i].q_bValue | BOOL | Yes | Array of digital output signals |
arrAO[i].q_nValue | INT | Yes | Array of analog output signals |
GUI Template¶
The IODev Library provides a template GUI GUI_TEMPLATE_IODEV for FB_IODEV_BASE FB. The GUI can be also used for the Function Blocks that extend the FB_IODEV_BASE functionality. Applications can easily deploy an instance of this GUI by setting the GUI references to the particular instance of the FB, as shown below.
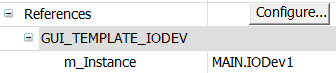
Instantiation of GUI_TEMPLATE_IODEV for IODev1

FB_IODEV_BASE HMI for Local Control.
IODev specific RPC Methods¶
- RPC_SetOutputs() Activate IODev outputs (not used with pure sensors)
Signal Simulators¶
The IODev.library provides individual analog and digital signal simulators. This means that if the user wants to simulate all signals of a sensor with eight digital and four analog signals, for example, he will have to instantiate eight digital and four analog signal simulators. However, for testing purposes it might be needed to simulate just a few signals. Time parameters are given in milliseconds.
The function block FB_SIM_SIGNAL_ANALOG implements a simulated analog sinusoidal signal. The input parameters are:
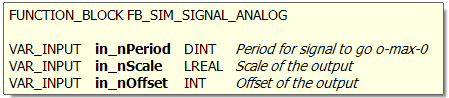
The function block FB_SIM_SIGNAL_DIGITAL implements a simulated digital signal. The input parameters are:

The following code example is the screen capture of the Program MAIN that is delivered with the library. The code shows how to configure sensors as well as simulators whose outputs could be mapped to sensor input variables.
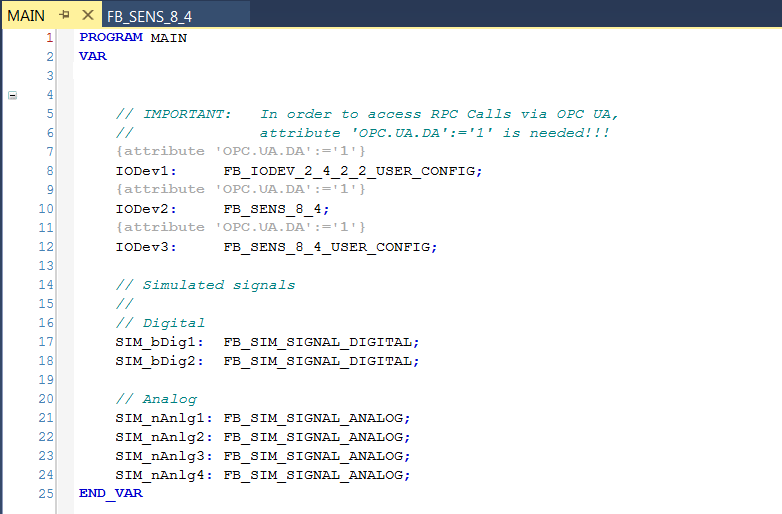
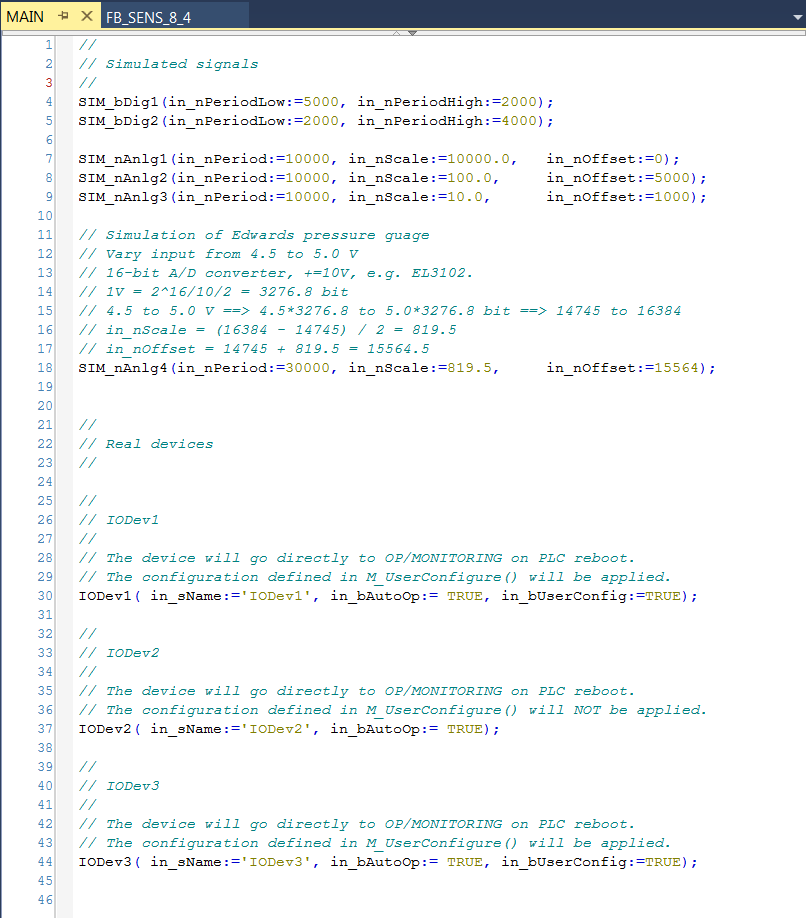
Simulator Mapping¶
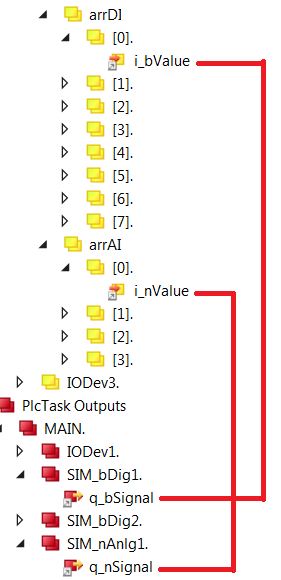
Communication Libraries (rsComm*.library)¶
Module rsComm contains PLC libraries for communication via serial port, Ethernet Tcp and Ethernet Tcp RT (Ethernet Tcp Real-Time). The libraries are delivered under separate PLC projects as part of the rsComm TwinCAT solution. The reason for this is that each communication protocol requires a separate license, so the functionality is provided per license.
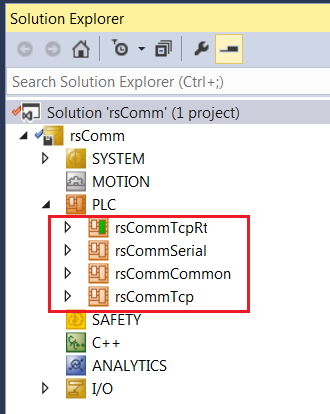
The table below gives the overview of the libraries.
Variable | Description |
---|---|
rsCommCommon | Common library that has to be included in every project using rsComm* libraries |
rsCommSerial | Serial port communication and modbus RTU |
rsCommTcp | TCP/IP communication via EtherCAT switch ports EL6601 and EL6614 |
rsCommTcpRt | TCP/IP Real-Time communication via CX2500-0060 |
rsCommCommon.library¶
This is the common library that has to be included under References in all projects using rsComm* libraries. The Function Block FB_RS_BASE provides the common state machine for all controllers. All rsComm PLC controllers, Serial, Tcp and TcpRt, extend the functionality of this FB.
rsCommSerial.library¶
This library handles communication via serial line, e.g. via EL6001. In addition to the FBs handling ASCII serial (RS-232/422/485) and modbus RTU communication protocols, it provides controllers for the standard equipment used at ESO that have the serial port interface, like the Lakeshore 340 temperature controller or the ESO Cabinet Cooling Controller.
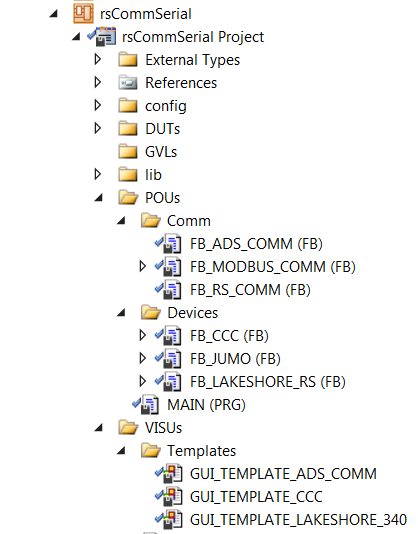
The overview of FBs is given in the table below.
Variable | Description |
---|---|
FB_RS_COMM | Generic RS-232/422/485 communication driver. |
FB_MODBUS_COMM | Generic modbus RTU communication driver via serial port. |
FB_CCC | ESO standard Cabinet Cooling Controller with RS-232 interface. |
FB_JUMO | JUMO controller with modbus RTU interface. |
FB_LAKESHORE_RS | Lakeshore driver for devices with serial port, i.e. models 218 & 340. |
Signal Mapping¶
Apart from the common i_nCouplerState input parameter that has to be mapped to any of the State variables on the PLC, the mapping has to be done for input and output parameters of the serial port.
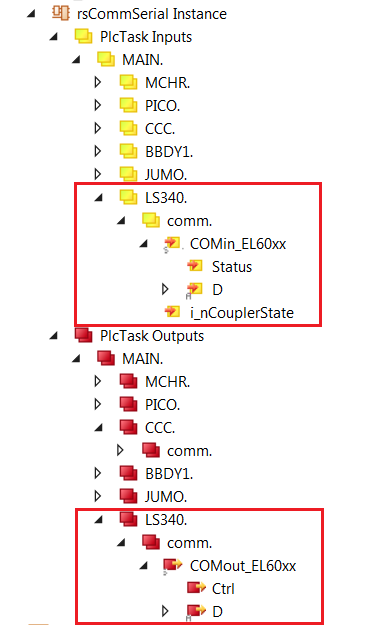
The serial port communication parameters, like baud rate, etc, have to be configured via the CoE interface.
The default CoE configuration for the Lakeshore 340 is given below.
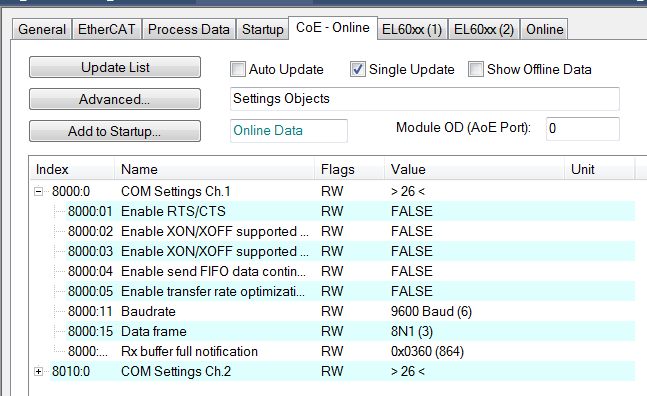
GUI Templates¶
GUI templates for the ESO Cabinet Cooling Controller and the Lakeshore 340 temperature controller are provided.
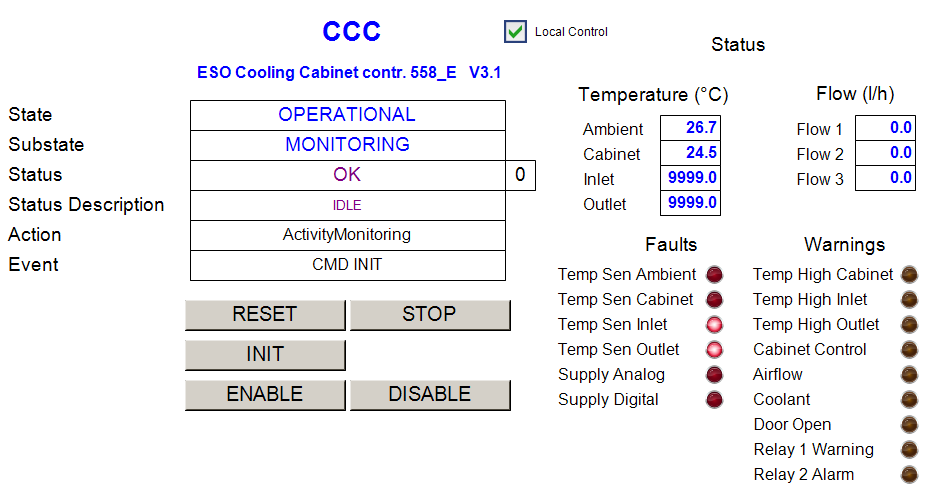
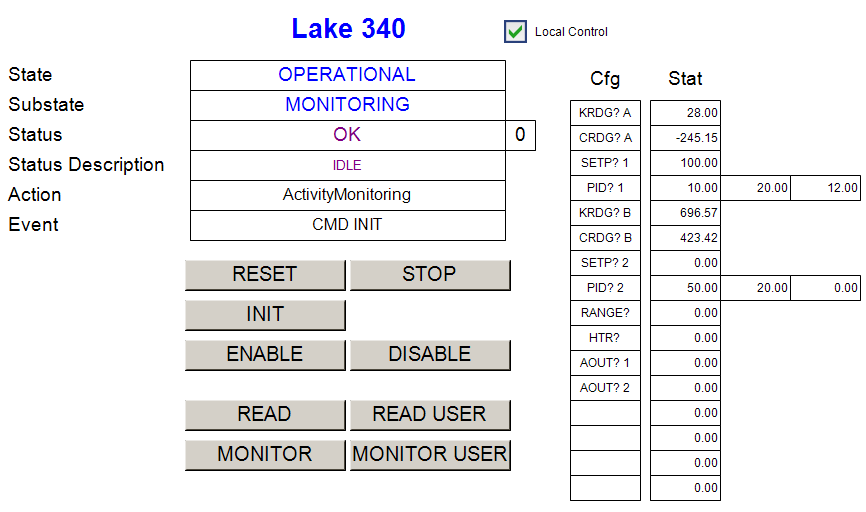
Example code¶
The following code handles an ESO Cabinet Cooling Controller, a JUMO controller and a Lakeshore 340.
PROGRAM MAIN
VAR
// ESO Cabinet Cooling Controller (RS-232 interface)
{attribute 'OPC.UA.DA' := '1'}
CCC: FB_CCC;
// JUMO Controller (modnus RTU via Serial)
{attribute 'OPC.UA.DA' := '1'}
JUMO: FB_JUMO;
// Lakeshore 340 (RS-232 interface)
{attribute 'OPC.UA.DA' := '1'}
LS340: FB_LAKESHORE_RS;
END_VAR
CCC(in_sName:='CCC', in_nPeriod := 1200);
JUMO(in_sName:='JUMO',in_nPeriod:=1000); // Read all JUMOs every second
LS340(in_sName :='Lake 340',
in_nModel :=340,
in_bAutoMonitor :=TRUE,
in_nPeriod :=1700);
rsCommTcp.library¶
Note
Usage of the rsCommTcp.library in combination with the EtherCAT switch ports EL6601 and EL6614 is the preferred option over the usage of the CX2500-0060 Realtime network adapter (see rsCommTcpRt.library).
This library handles the TCP/IP communication via EtherCAT switch ports EL6601 and EL6614. It can be used to communicate with devices equipment with the Ethernet port, e.g. the Lakeshore 336 temperature controller. It requires TF6310 TCP/IP license. In addition, the TF6310 function Windows driver has to be installed on the PLC. The IP address of the switch has to match the subnet of the device connected to it. For more info about installation and licensing consult Beckhoff documentation.
The library provides generic TCP/IP drivers for TCP clients (FB_TCP_CLIENT) and servers (FB_TCP_SERVER). Device PLC controllers are based on FB_TCP_CLIENT, while FB_TCP_SERVER is used only for simulators.
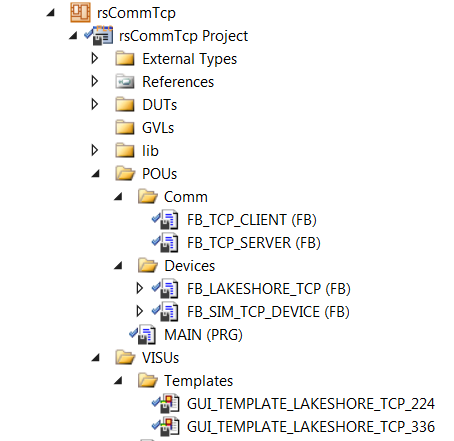
The overview of FBs is given in the table below.
Variable | Description |
---|---|
FB_TCP_CLIENT | Generic TCP/IP Client driver. |
FB_TCP_SERVER | Generic TCP/IP Server driver. |
FB_LAKESHORE_TCP | Lakeshore driver for devices with Ethernet port, i.e. models 224 & 336. |
FB_SIM_TCP_DEVICE | Generic TCP/IP device simulator with support for Lakeshore models 224 & 336. |
Signal Mapping¶
Devices and simulators have only a single input parameter (i_nCouplerState) to be mapped to any of the State variables on the PLC.
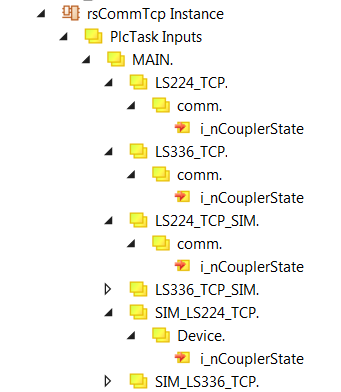
GUI Templates¶
GUI templates for the Lakeshore 336 and 224 temperature controller/monitor are provided. See GUI Templates under rsCommTcpRt.library.
Example code¶
The following code handles two real Lakeshore devices (224 and 336) and two additional Lakeshore devices that are connected to simulators. It is important to note that the devices that use simulators have to use the IP address ‘127.0.0.1’. Also, the port numbers of the device and the simulator have to match.
PROGRAM MAIN
VAR
// Lakeshore 224 (Ethernet interface)
{attribute 'OPC.UA.DA' := '1'}
LS224_TCP: FB_LAKESHORE_TCP; // LakeShore based on FB_TCP_CLIENT
// Lakeshore 336 (Ethernet interface)
{attribute 'OPC.UA.DA' := '1'}
LS336_TCP: FB_LAKESHORE_TCP; // LakeShore based on FB_TCP_CLIENT
// Lakeshores to be simulated
{attribute 'OPC.UA.DA' := '1'}
LS224_TCP_SIM: FB_LAKESHORE_TCP; // LakeShore based on FB_TCP_CLIENT
{attribute 'OPC.UA.DA' := '1'}
LS336_TCP_SIM: FB_LAKESHORE_TCP; // LakeShore based on FB_TCP_CLIENT
// Simulators
SIM_LS224_TCP: FB_SIM_TCP_DEVICE; // LakeShore SIM based on FB_TCP_SERVER
SIM_LS336_TCP: FB_SIM_TCP_DEVICE; // LakeShore SIM based on FB_TCP_SERVER
END_VAR
// Lakeshore 224
LS224_TCP( in_sName := 'LS224_TCP',
in_nModel := 224,
in_nPeriod := 1500,
in_sCmdSuffix := '$0D$0A',
in_sDeviceTcpIpAdr := '192.168.0.12',
in_nDeviceTcpPort := 7777);
// Lakeshore 336
LS336_TCP( in_sName := 'LS336_TCP',
in_nModel := 336,
in_nPeriod := 1000,
in_sCmdSuffix := '$0A',
in_sDeviceTcpIpAdr := '192.168.0.80',
in_nDeviceTcpPort := 7777);
// Dummy Lakeshore 224
LS224_TCP_SIM( in_sName := 'LS224_TCP_SIM',
in_nModel := 224,
in_nPeriod := 1500,
in_sCmdSuffix := '$0D$0A',
in_sDeviceTcpIpAdr := '127.0.0.1',
in_nDeviceTcpPort := 8888);
// Dummy Lakeshore 336
LS336_TCP_SIM( in_sName := 'LS336_TCP_SIM',
in_nModel := 336,
in_nPeriod := 1000,
in_sCmdSuffix := '$0A',
in_sDeviceTcpIpAdr := '127.0.0.1',
in_nDeviceTcpPort := 7777);
// Lakeshore simulators
SIM_LS224_TCP( in_bEnable:= TRUE,
in_nDeviceType:=E_TCP_DEVICE_TYPE.LAKESHORE_224,
in_sTcpIpAdr:='127.0.0.1',
in_nTcpPort:=8888);
SIM_LS336_TCP( in_bEnable:= TRUE,
in_nDeviceType:=E_TCP_DEVICE_TYPE.LAKESHORE_336,
in_sTcpIpAdr:='127.0.0.1',
in_nTcpPort:=7777);
rsCommTcpRt.library¶
This library handles the TCP/UDP communication via CX2500-0060 system module that provides two independent Gbit Ethernet interfaces to the CX2000 family of PLCs, e.g. CX2030. The library can be used to communicate with devices equipment with the Ethernet port, e.g. the Lakeshore 336 temperature controller. It requires TF6311 TC3 TCP/UDP Realtime license. The IP address of the switch has to match the subnet of the device connected to it. For more info about installation and licensing consult Beckhoff documentation.
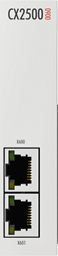
Warning
If the CX2500-0060 system module is installed, the PCI Bus address of the EtherCAT Device will be changed from the default 4/0 (0xF0020000) to 8/0 (0xF0020000), and an attempt to download an existing project will result in a failure message that is impossible to understand. For new projects the system will recognise the address automatically, while for the existing ones the new address has to be set by pressing the ‘Search…’ button and selecting the only available PCI Bus address as seen on the figure below.
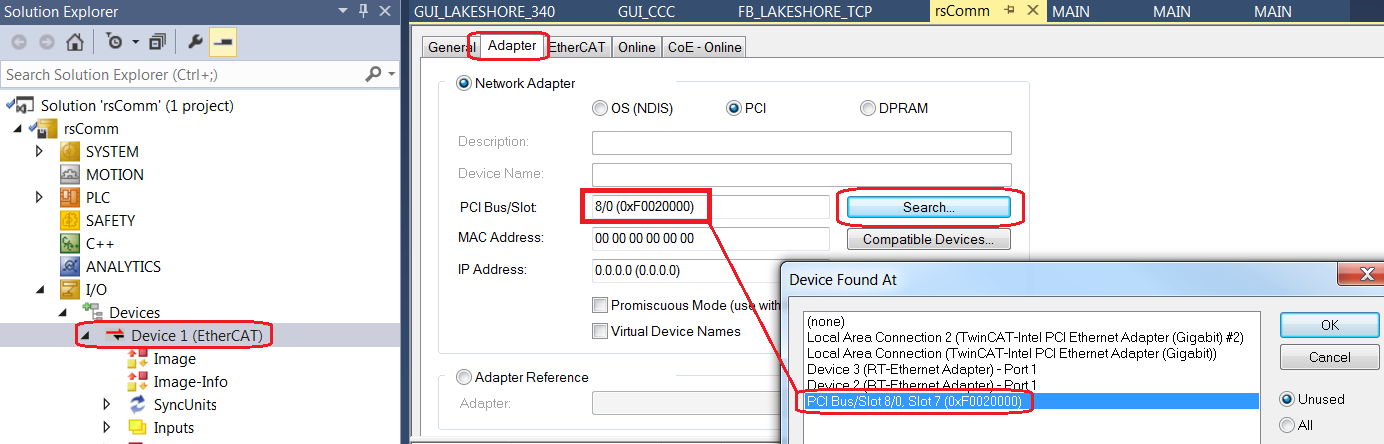
The library provides a generic TCP/UDP RT driver for TCP RT clients (FB_TCP_RT_CLIENT) that is used in PLC controllers for the equipment with the Ethernet interface, e.g. the Lakeshore 336.
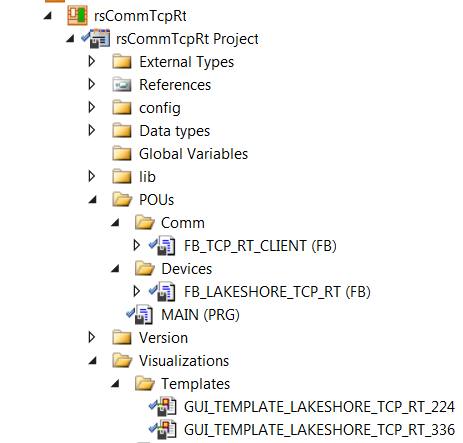
The overview of FBs is given in the table below.
Variable | Description |
---|---|
FB_TCP_RT_CLIENT | Generic TCP/UDP RT Client driver. |
FB_LAKESHORE_TCP_RT | Lakeshore driver for devices with Ethernet port, i.e. models 224 & 336. |
Adapter Configuration¶
The CX2500-0060 system module is not part of the EtherCAT network and there is no ‘clasical’ EtherCAT I/O mapping. Each network port has to be added manually under the I/O Devices as an RT-Ethernet Adapter. Then, an Object of type TCP/UDP RT, that can be found under Beckhoff/TcIoEth Modules, has to be added to the adaptor, e.g. Device 2 (RT-Ethernet Adapter)_Obj1 (TCP/UDP RT).
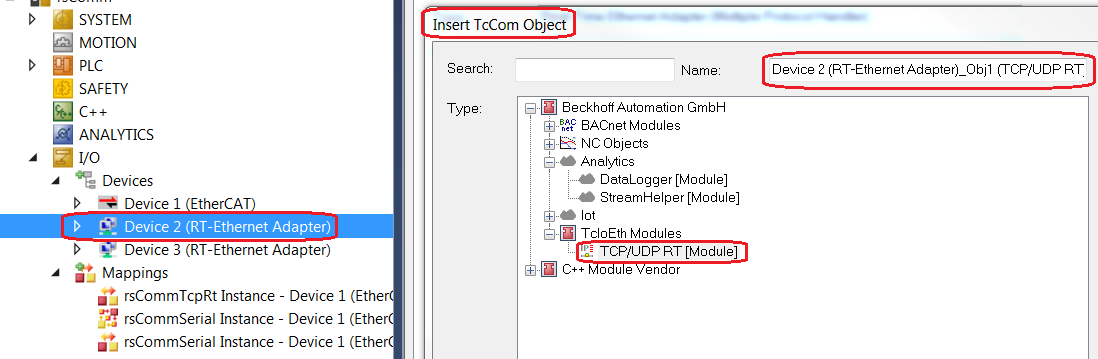
The Context of the Object is the PLC Task where an instance of the devices, e.g. MAIN.LS224_TCP_RT, will be running.

In the ‘Symbol Initialisation’ the Object has to be linked to the device instance.
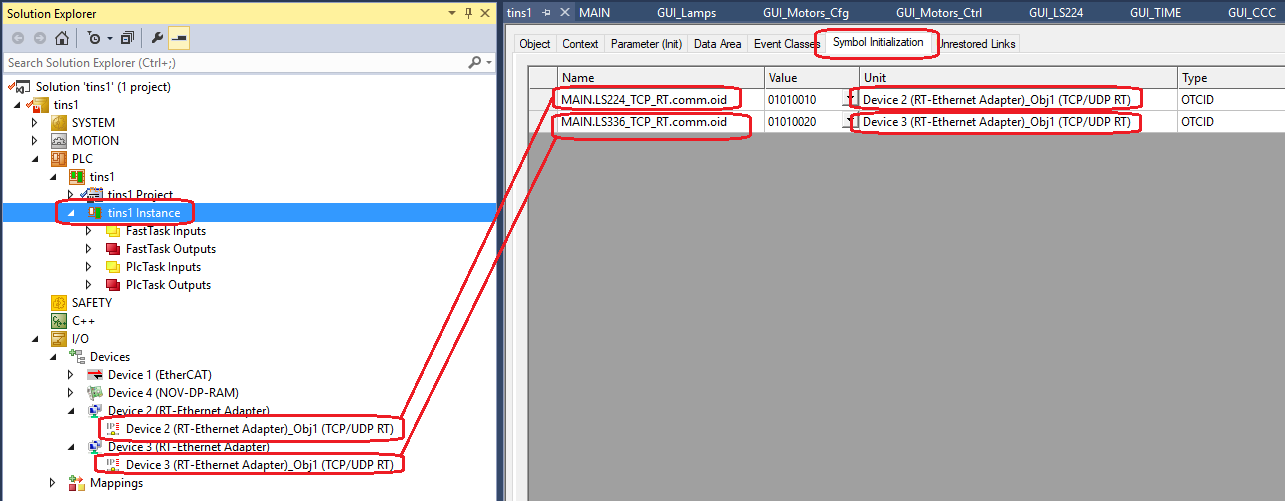
The ‘Interface Pointer’ has to be set to the Device Object.
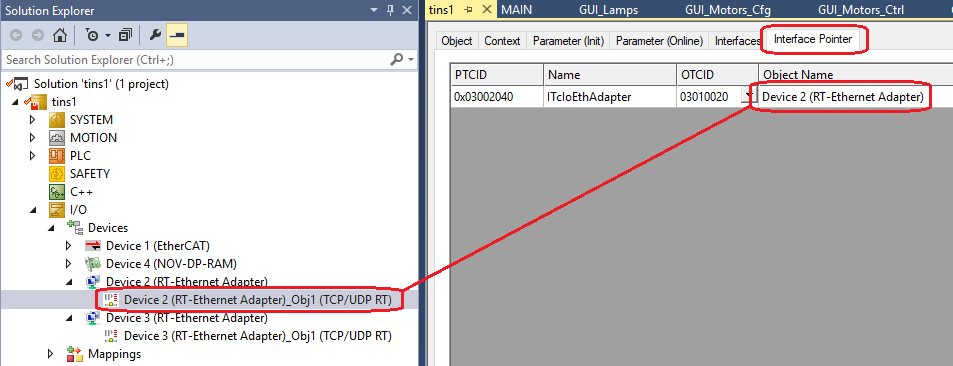
In the case of a Lakeshore device connected to the RT-EthernetAdapter Object, the TcpTimeoutIdle, that can be found under ‘Parameter (Init)’, has to be set to 30 ms. This is a Lakeshore specific setting.
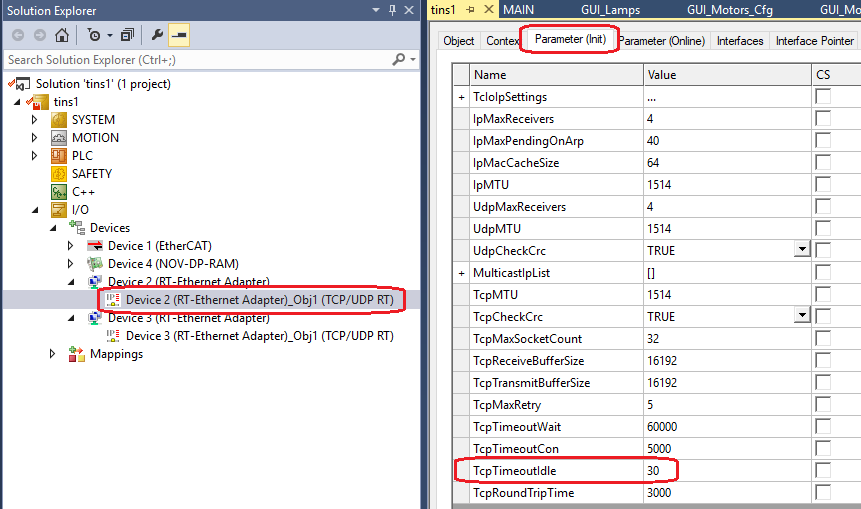
GUI Templates¶
GUI templates for the Lakeshore 336 and 224 temperature controller/monitor are provided.
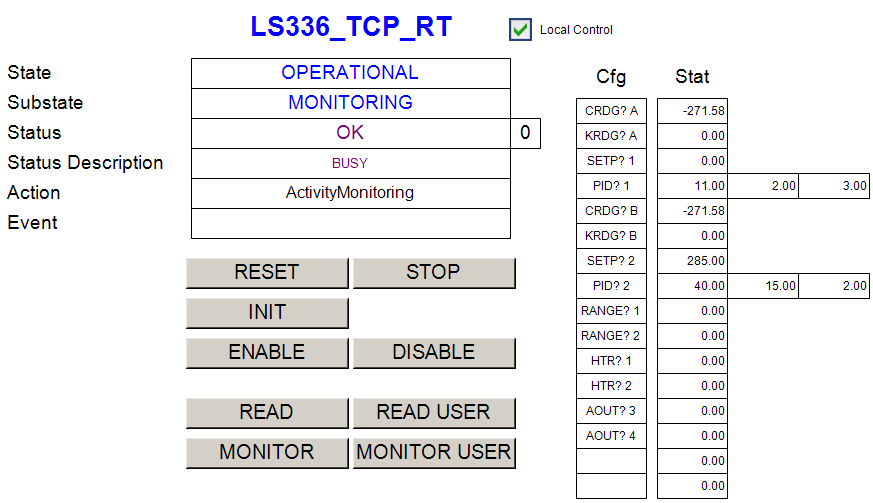
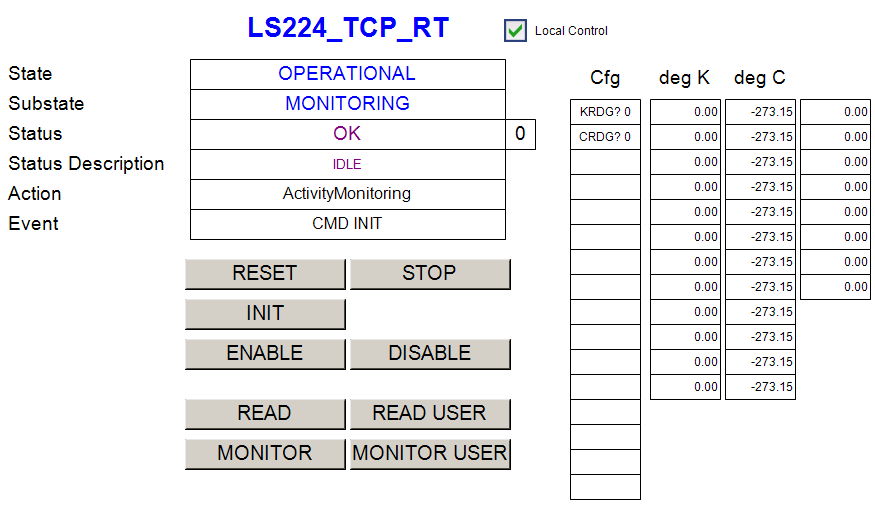
Example code¶
The following code handles two Lakeshore devices (224 and 336).
PROGRAM MAIN
VAR
//
// Tcp RT interface functions
//
// Lakeshore 224 (Ethernet TCP Realtime interface)
{attribute 'OPC.UA.DA' := '1'}
LS224_TCP_RT: FB_LAKESHORE_TCP_RT; // LakeShore based on FB_TCP_RT_CLIENT
// Lakeshore 336 (Ethernet TCP Realtime interface)
{attribute 'OPC.UA.DA' := '1'}
LS336_TCP_RT: FB_LAKESHORE_TCP_RT; // LakeShore based on FB_TCP_RT_CLIENT
END_VAR
//
// Tcp RT interface functions
//
// Read every 2000 ms
// Command terminator for Lakeshore 224 is '$0D$0A'
LS224_TCP_RT(in_sName := 'LS224_TCP_RT',
in_nModel := 224,
in_sCmdSuffix := '$0D$0A',
in_sDeviceTcpIpAdr := '192.168.0.12',
in_nDeviceTcpPort := 7777,
in_nPeriod := 2000);
// Read every 1000 ms
// Command terminator for Lakeshore 336 is '$0A'
LS336_TCP_RT(in_sName := 'LS336_TCP_RT',
in_nModel := 336,
in_sCmdSuffix := '$0A',
in_sDeviceTcpIpAdr := '192.168.0.80',
in_nDeviceTcpPort := 7777,
in_nPeriod := 1000);
Piezo Library (Piezo.library)¶
The Piezo.library provides a generic PLC Piezo controller FB FB_PIEZO_BASE that has to be customised (extended) by the user. Up to three output control signals of type INT are supported (configurable). The example FB called FB_PIEZO_EXAMPLE shows how this customisation could be done.
The figure below shows what is delivered in the library.
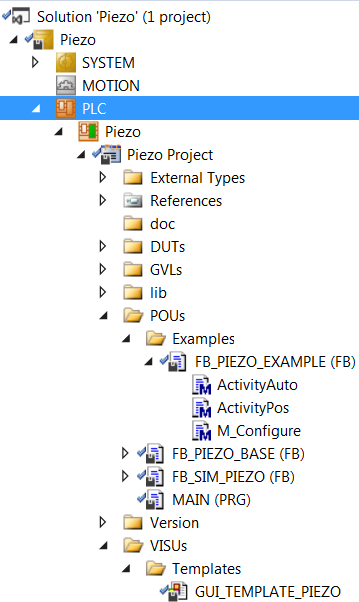
Input parameters¶

Signal Mapping¶
The figure below shows the TwinCAT view of the FB_PIEZO_BASE I/O variables that are available for mapping to physical signals, i.e. ports of I/O terminals.
There are two arrays for the feedback signals, one of type INT and the other one of type DINT. Any of them can be used in the customised application. There is an array for the control output signals of type INT.
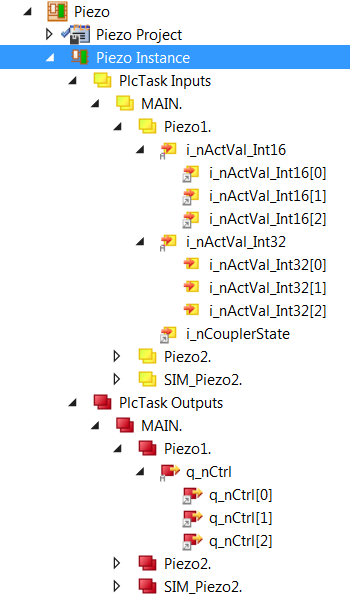
The table below describes each mapping variable.
Variable | Port Type | Optional Mapping | Description |
---|---|---|---|
i_nCouplerState | UINT | No | Mapped to the ‘state’ of the coupler that hosts I/O terminals. If the terminals span over more than one coupler, it is recommended to select the ‘state’ of the last coupler that hosts a lamp signal. |
i_nActVal_Int16 | INT | Yes | Array [0..2] of feedback signals of type INT |
i_nActVal_Int32 | DINT | Yes | Array [0..2] of feedback signals of type DINT |
q_nCtrl | INT | No | Array [0..2] of control output signals of type INT |
GUI Template¶
The Piezo.library provides a template GUI for the control of Piezo devices. Applications can easily deploy an instance of this GUI by setting the GUI references to a particular instance, as shown below.

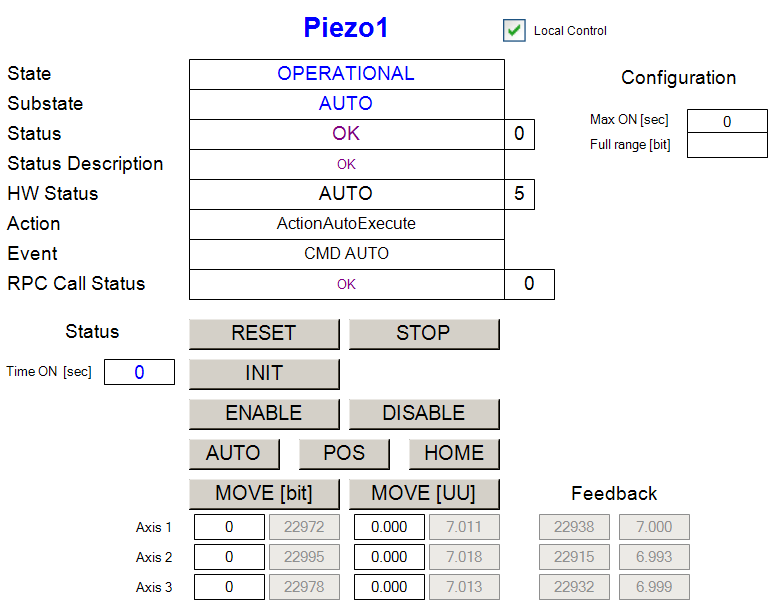
Piezo specific RPC Methods¶
Method | Description |
---|---|
RPC_Auto | Start Automatic Loop. The user has to overload method ActivityAuto() |
RPC_Home | Move piezos to Home position |
RPC_MoveBit | Move piezos to positions given in [bit] |
RPC_MoveUser | Move piezos to positions given in user units [UU] |
RPC_Pos | Keep the piezos where they are (default behaviour). This might mean to stop the Auto loop. The user can overload this method. |
Piezo Simulator¶
The function block FB_SIM_PIEZO implements the piezo simulator on the PLC. The FB doesn’t have any input parameter. The simulator takes the real piezo device control outputs and generate its own feedback output signals by adding sine wave offsets. The simulator output feedback is mapped to the feedback inputs of the simulated device. The sine wave is fully configurable (amplitude and cycle period).
Piezo Simulator RPC Methods¶
Method | Description |
---|---|
RPC_ResetConfig | Reset simulator configuration to its default. |
RPC_SetCouplerState | Set coupler state. Any value other than 8 would cause a failure of the simulated device. |
RPC_SetMaxError | Set Maximal Simulated feedback offset [bit] and the Period for complete sine wave offset cycle [msec] |
Simulator Mapping¶
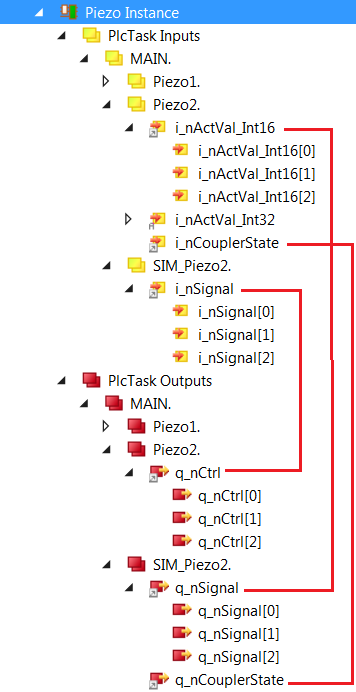
Sample Code¶
The following code represent a real Piezo controller (Piezo1) and a piezo controller (Piezo2) that is connected to a simulator (SIM_Piezo2). The mapping is shown in the Simulator Mapping section.
PROGRAM MAIN
VAR
{attribute 'OPC.UA.DA':='1'}
Piezo1: FB_PIEZO_EXAMPLE; // Piezo #1
{attribute 'OPC.UA.DA':='1'}
Piezo2: FB_PIEZO_EXAMPLE; // Piezo #2
{attribute 'OPC.UA.DA':='1'}
SIM_Piezo2: FB_SIM_PIEZO; // Simulator for Piezo #2
END_VAR
Piezo1(in_sName:='Piezo1', in_nNumAxes:=3);
Piezo2(in_sName:='Piezo2', in_nNumAxes:=3);
SIM_Piezo2();